安卓代码通过libssh.so实现ssh端口转发
时间: 2023-07-25 17:24:05 浏览: 238
在安卓应用程序中使用libssh.so实现ssh端口转发,可以通过以下步骤实现:
1. 在app的build.gradle文件中添加以下代码,引入libssh.so库:
```gradle
android {
// ...
sourceSets.main {
jniLibs.srcDirs = ['libs']
}
}
```
将libssh.so库复制到app/libs目录下。
2. 在需要进行端口转发的地方,调用以下代码:
```java
// 初始化ssh会话
SshSession session = new SshSession("172.200.37.37", "cts-android", "Stb.20230128", 22);
session.connect();
// 进行端口转发
int remotePort = 5556;
int localPort = 5555;
session.portForward(remotePort, localPort);
// 关闭ssh会话
session.disconnect();
```
其中,SshSession是一个自定义的类,用于封装libssh.so的功能。其中包含了连接远程服务器、进行端口转发、关闭ssh会话等方法的实现。
SshSession类的代码如下:
```java
public class SshSession {
private static final String TAG = "SshSession";
private String host;
private String username;
private String password;
private int port;
private ssh_session session;
private ssh_channel channel;
public SshSession(String host, String username, String password, int port) {
this.host = host;
this.username = username;
this.password = password;
this.port = port;
}
// 连接ssh服务器
public void connect() {
int rc;
// 创建ssh会话
session = ssh_new();
// 设置ssh会话参数
ssh_options_set(session, SSH_OPTIONS_HOST, host);
ssh_options_set(session, SSH_OPTIONS_USER, username);
ssh_options_set(session, SSH_OPTIONS_PORT, port);
// 连接ssh服务器
rc = ssh_connect(session);
if (rc != SSH_OK) {
Log.e(TAG, "Unable to connect to host " + host + ":" + port + ": " + ssh_get_error(session));
return;
}
// 鉴权
rc = ssh_userauth_password(session, NULL, password);
if (rc != SSH_AUTH_SUCCESS) {
Log.e(TAG, "Authentication failed: " + ssh_get_error(session));
return;
}
Log.i(TAG, "Connected to host " + host + ":" + port);
}
// 进行端口转发
public void portForward(int remotePort, int localPort) {
int rc;
// 创建通道
channel = ssh_channel_new(session);
if (channel == null) {
Log.e(TAG, "Failed to create channel: " + ssh_get_error(session));
return;
}
// 开始通道转发
rc = ssh_channel_open_forward(channel, "127.0.0.1", localPort, "127.0.0.1", remotePort);
if (rc != SSH_OK) {
Log.e(TAG, "Failed to open channel: " + ssh_get_error(session));
return;
}
Log.i(TAG, "Port forward " + remotePort + " -> " + localPort + " started");
}
// 关闭ssh会话
public void disconnect() {
if (channel != null) {
ssh_channel_free(channel);
}
if (session != null) {
ssh_disconnect(session);
ssh_free(session);
}
Log.i(TAG, "Disconnected from host " + host + ":" + port);
}
}
```
在connect方法中,创建ssh会话并连接到远程服务器。在portForward方法中,创建ssh通道并进行端口转发。在disconnect方法中,关闭ssh会话。
需要注意的是,在使用libssh.so库进行ssh连接时,需要在应用程序的AndroidManifest.xml文件中添加以下权限:
```xml
<uses-permission android:name="android.permission.INTERNET" />
```
这是因为ssh连接需要通过网络进行通信。
阅读全文
相关推荐
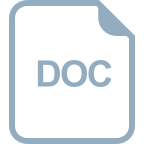
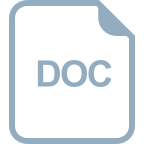
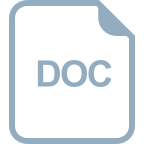

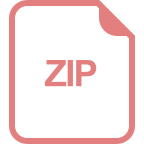





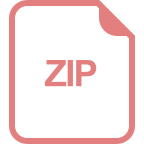






