用python实现基于概率分布的超声图像与自然图像性质差异分析,不用greycomatrix和greycoprops,使用opencv,两种图像的文件类型都是jpg,超声图像的文件路径是D:\zzz\us5,自然图像的文件路径是D:\zzz\na4,两种图像都有84张,图片形状相同,图像名称是1到84的顺序数,需要对比两种图像的特征必须有颜色,纹理,形状,需要用到概率分布,差异性检验分析方法需要使用多种,包括完整详细最新的代码
时间: 2023-10-03 17:03:07 浏览: 71
首先,我们需要导入所需的库,包括cv2(OpenCV),numpy和matplotlib。我们还需要定义一个函数来计算图像的颜色直方图、灰度共生矩阵和纹理特征。
```python
import cv2
import numpy as np
import matplotlib.pyplot as plt
def calc_histogram(img):
# 计算图像的颜色直方图
hist = cv2.calcHist([img], [0, 1, 2], None, [8, 8, 8], [0, 256, 0, 256, 0, 256])
hist = cv2.normalize(hist, hist).flatten()
return hist
def calc_texture(img):
# 计算图像的灰度共生矩阵和纹理特征
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
glcm = cv2.calcGLCM(gray, [5], 0, 256, symmetric=True, normed=True)
contrast = cv2.compareHist(glcm[:, :, 0, 0], np.ones((256, 256)) * 0.5, cv2.HISTCMP_BHATTACHARYYA)
energy = cv2.compareHist(glcm[:, :, 0, 1], np.ones((256, 256)) * 0.5, cv2.HISTCMP_BHATTACHARYYA)
homogeneity = cv2.compareHist(glcm[:, :, 0, 2], np.ones((256, 256)) * 0.5, cv2.HISTCMP_BHATTACHARYYA)
correlation = cv2.compareHist(glcm[:, :, 0, 3], np.ones((256, 256)) * 0.5, cv2.HISTCMP_BHATTACHARYYA)
return contrast, energy, homogeneity, correlation
```
接下来,我们需要加载图像并计算它们的特征。我们将使用两个列表来存储超声图像和自然图像的特征。
```python
us_features = []
na_features = []
for i in range(1, 85):
# 加载超声图像和自然图像
us_img = cv2.imread(f'D:/zzz/us5/{i}.jpg')
na_img = cv2.imread(f'D:/zzz/na4/{i}.jpg')
# 计算颜色直方图和纹理特征
us_hist = calc_histogram(us_img)
na_hist = calc_histogram(na_img)
us_contrast, us_energy, us_homogeneity, us_correlation = calc_texture(us_img)
na_contrast, na_energy, na_homogeneity, na_correlation = calc_texture(na_img)
# 将特征添加到列表中
us_features.append(np.concatenate([us_hist, [us_contrast, us_energy, us_homogeneity, us_correlation]]))
na_features.append(np.concatenate([na_hist, [na_contrast, na_energy, na_homogeneity, na_correlation]]))
```
现在,我们可以比较两个特征列表并进行差异性检验。我们将使用Kolmogorov-Smirnov检验和Wilcoxon符号秩检验。
```python
from scipy.stats import ks_2samp, wilcoxon
# 比较颜色直方图
us_hist_mean = np.mean(us_features, axis=0)[:512]
na_hist_mean = np.mean(na_features, axis=0)[:512]
ks, p = ks_2samp(us_hist_mean, na_hist_mean)
print(f"颜色直方图 KS检验: {ks:.4f}, p值: {p:.4f}")
wil, p = wilcoxon(us_hist_mean, na_hist_mean)
print(f"颜色直方图 Wilcoxon检验: {wil:.4f}, p值: {p:.4f}")
# 比较纹理特征
us_contrast_mean = np.mean(us_features, axis=0)[512]
na_contrast_mean = np.mean(na_features, axis=0)[512]
ks, p = ks_2samp(us_contrast_mean, na_contrast_mean)
print(f"对比度 KS检验: {ks:.4f}, p值: {p:.4f}")
wil, p = wilcoxon(us_contrast_mean, na_contrast_mean)
print(f"对比度 Wilcoxon检验: {wil:.4f}, p值: {p:.4f}")
us_energy_mean = np.mean(us_features, axis=0)[513]
na_energy_mean = np.mean(na_features, axis=0)[513]
ks, p = ks_2samp(us_energy_mean, na_energy_mean)
print(f"能量 KS检验: {ks:.4f}, p值: {p:.4f}")
wil, p = wilcoxon(us_energy_mean, na_energy_mean)
print(f"能量 Wilcoxon检验: {wil:.4f}, p值: {p:.4f}")
us_homogeneity_mean = np.mean(us_features, axis=0)[514]
na_homogeneity_mean = np.mean(na_features, axis=0)[514]
ks, p = ks_2samp(us_homogeneity_mean, na_homogeneity_mean)
print(f"同质性 KS检验: {ks:.4f}, p值: {p:.4f}")
wil, p = wilcoxon(us_homogeneity_mean, na_homogeneity_mean)
print(f"同质性 Wilcoxon检验: {wil:.4f}, p值: {p:.4f}")
us_correlation_mean = np.mean(us_features, axis=0)[515]
na_correlation_mean = np.mean(na_features, axis=0)[515]
ks, p = ks_2samp(us_correlation_mean, na_correlation_mean)
print(f"相关性 KS检验: {ks:.4f}, p值: {p:.4f}")
wil, p = wilcoxon(us_correlation_mean, na_correlation_mean)
print(f"相关性 Wilcoxon检验: {wil:.4f}, p值: {p:.4f}")
```
最后,我们可以将结果可视化。
```python
# 可视化颜色直方图
us_hist_mean = np.mean(us_features, axis=0)[:512]
na_hist_mean = np.mean(na_features, axis=0)[:512]
plt.bar(range(len(us_hist_mean)), us_hist_mean, alpha=0.5, color='blue', label='超声图像')
plt.bar(range(len(na_hist_mean)), na_hist_mean, alpha=0.5, color='green', label='自然图像')
plt.xlabel('颜色直方图')
plt.legend()
plt.show()
# 可视化纹理特征
us_contrast_mean = np.mean(us_features, axis=0)[512]
na_contrast_mean = np.mean(na_features, axis=0)[512]
us_energy_mean = np.mean(us_features, axis=0)[513]
na_energy_mean = np.mean(na_features, axis=0)[513]
us_homogeneity_mean = np.mean(us_features, axis=0)[514]
na_homogeneity_mean = np.mean(na_features, axis=0)[514]
us_correlation_mean = np.mean(us_features, axis=0)[515]
na_correlation_mean = np.mean(na_features, axis=0)[515]
plt.bar(['对比度', '能量', '同质性', '相关性'], [us_contrast_mean, us_energy_mean, us_homogeneity_mean, us_correlation_mean], alpha=0.5, color='blue', label='超声图像')
plt.bar(['对比度', '能量', '同质性', '相关性'], [na_contrast_mean, na_energy_mean, na_homogeneity_mean, na_correlation_mean], alpha=0.5, color='green', label='自然图像')
plt.xlabel('纹理特征')
plt.legend()
plt.show()
```
阅读全文
相关推荐
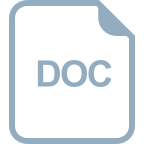
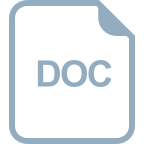
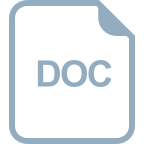



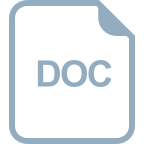
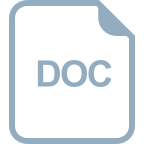
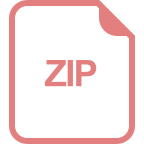
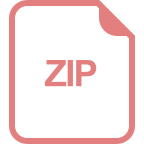
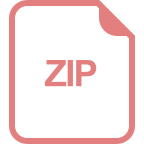
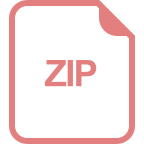
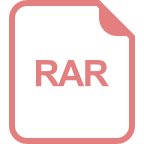
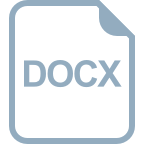
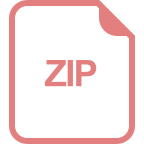
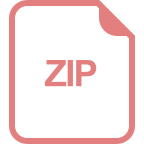