c语言读取并显示jpg图片
时间: 2023-12-02 19:03:11 浏览: 55
要在C语言中读取并显示JPG图片,需要使用第三方库来处理。其中,比较常用的库包括libjpeg和OpenCV。
使用libjpeg库可以按照以下步骤进行:
1. 下载并安装libjpeg库;
2. 在代码中引入jpeglib.h头文件,定义相应的结构体和变量,如下所示:
```
#include <stdio.h>
#include <jpeglib.h>
struct jpeg_decompress_struct cinfo;
struct jpeg_error_mgr jerr;
JSAMPROW row_pointer[1];
```
3. 打开JPG图片文件,并将其解压缩,如下所示:
```
FILE *infile = fopen("example.jpg", "rb");
if (!infile) {
printf("Error opening jpeg file");
return 0;
}
cinfo.err = jpeg_std_error(&jerr);
jpeg_create_decompress(&cinfo);
jpeg_stdio_src(&cinfo, infile);
jpeg_read_header(&cinfo, TRUE);
jpeg_start_decompress(&cinfo);
```
4. 读取图片像素数据,并将其存储到数组中,如下所示:
```
int row_stride = cinfo.output_width * cinfo.output_components;
unsigned char *buffer = new unsigned char[row_stride * cinfo.output_height];
while (cinfo.output_scanline < cinfo.output_height) {
row_pointer[0] = &buffer[(cinfo.output_height - cinfo.output_scanline - 1) * row_stride];
jpeg_read_scanlines(&cinfo, row_pointer, 1);
}
```
5. 关闭文件并释放资源,如下所示:
```
jpeg_finish_decompress(&cinfo);
jpeg_destroy_decompress(&cinfo);
fclose(infile);
```
至此,JPG图片的像素数据已经存储在了buffer数组中。接下来,可以使用其他图像库或工具来显示或处理该图像数据。
相关推荐
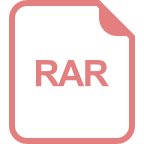













