Using the mbed development environment and Freescale FRDM-KL25Z, the lamp brightness is adjusted using keys and three colors are displayed for brightness.it should detailed code comments
时间: 2024-03-05 12:52:02 浏览: 18
Sure, I can help you with that! Here's an example code for adjusting the brightness of an RGB LED using the keys on the Freescale FRDM-KL25Z board. The code is written in C++ using the mbed development environment:
```
#include "mbed.h"
// Define pins for the RGB LED
PwmOut red(LED_RED);
PwmOut green(LED_GREEN);
PwmOut blue(LED_BLUE);
// Define pins for the keys
DigitalIn key1(PTC6);
DigitalIn key2(PTC7);
// Define the initial brightness values
float brightness = 0.5f;
float redBrightness = 0.5f;
float greenBrightness = 0.5f;
float blueBrightness = 0.5f;
int main() {
// Set the PWM frequency for the RGB LED
red.period(0.001f);
green.period(0.001f);
blue.period(0.001f);
while(1) {
// Check if key 1 is pressed
if (key1 == 0) {
// Increase the brightness
brightness += 0.1f;
if (brightness > 1.0f) {
brightness = 1.0f;
}
}
// Check if key 2 is pressed
if (key2 == 0) {
// Decrease the brightness
brightness -= 0.1f;
if (brightness < 0.0f) {
brightness = 0.0f;
}
}
// Update the red brightness
redBrightness = brightness;
// Update the green brightness
greenBrightness = 1.0f - brightness;
// Update the blue brightness
blueBrightness = fabs(brightness - 0.5f) * 2.0f;
// Set the PWM duty cycle for the RGB LED
red.write(redBrightness);
green.write(greenBrightness);
blue.write(blueBrightness);
// Wait for a short time
wait(0.1f);
}
}
```
Let me explain the code in detail:
- We first define the pins for the RGB LED and the keys using the `PwmOut` and `DigitalIn` classes from the mbed library.
- We then define the initial brightness values for the RGB LED, which are all set to 50%.
- In the `main()` function, we set the PWM frequency for the RGB LED and start an infinite loop.
- Inside the loop, we check if the first key is pressed. If it is, we increase the brightness by 10% and make sure it doesn't go above 100%. If the second key is pressed, we decrease the brightness by 10% and make sure it doesn't go below 0%.
- We then calculate the new brightness values for the red, green, and blue channels based on the overall brightness value. The red brightness is set to the overall brightness, the green brightness is set to the complement of the overall brightness, and the blue brightness is set to a scaled version of the difference between the overall brightness and 50%.
- Finally, we set the PWM duty cycles for the red, green, and blue channels using the `write()` method of the `PwmOut` class, and wait for a short time before starting the loop again.
I hope this helps! Let me know if you have any questions.
相关推荐
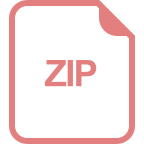
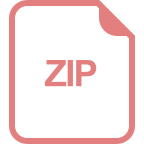
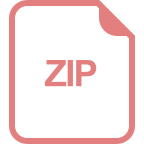














