Java nio使用例子
时间: 2023-07-11 09:23:03 浏览: 69
下面是一个简单的 Java NIO 示例,展示了如何使用 NIO 读取文件:
```java
import java.io.IOException;
import java.nio.ByteBuffer;
import java.nio.channels.FileChannel;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.StandardOpenOption;
public class NioExample {
public static void main(String[] args) {
// 定义文件路径
Path path = Paths.get("path/to/file.txt");
try (FileChannel fileChannel = FileChannel.open(path, StandardOpenOption.READ)) {
// 创建缓冲区
ByteBuffer buffer = ByteBuffer.allocate(1024);
// 从通道中读取数据
while (fileChannel.read(buffer) > 0) {
// 反转缓冲区,准备读取数据
buffer.flip();
// 将缓冲区中的数据转换为字符串并打印输出
System.out.print(new String(buffer.array(), 0, buffer.limit()));
// 清空缓冲区,准备写入数据
buffer.clear();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在以上示例中,我们首先定义了要读取的文件路径,然后使用 `FileChannel` 打开文件通道。接着,我们创建了一个 `ByteBuffer` 缓冲区,用于存储从文件中读取的数据。在 while 循环中,我们不断从通道中读取数据,并将读取的数据写入缓冲区。每次读取完后,我们需要反转缓冲区以便读取数据,并将缓冲区中的数据转换为字符串输出。最后,我们将缓冲区清空,以便写入下一批数据。
相关推荐
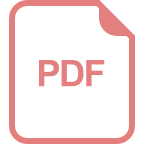
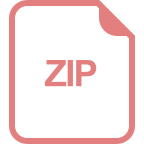














