js获取来访用户的ip并输出到页面
时间: 2023-08-31 15:39:35 浏览: 171
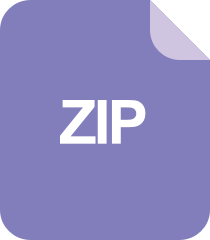
获得访问者的IP地址
### 回答1:
为了获取来访用户的 IP 地址,你可以使用以下 JavaScript 代码:
```
function getUserIP(onNewIP) { // onNewIp - your listener function for new IPs
//compatibility for firefox and chrome
var myPeerConnection = window.RTCPeerConnection || window.mozRTCPeerConnection || window.webkitRTCPeerConnection;
var pc = new myPeerConnection({
iceServers: []
}),
noop = function() {},
localIPs = {},
ipRegex = /([0-9]{1,3}(\.[0-9]{1,3}){3}|[a-f0-9]{1,4}(:[a-f0-9]{1,4}){7})/g,
key;
function iterateIP(ip) {
if (!localIPs[ip]) onNewIP(ip);
localIPs[ip] = true;
}
//create a bogus data channel
pc.createDataChannel("");
// create offer and set local description
pc.createOffer(function(sdp) {
sdp.sdp.split('\n').forEach(function(line) {
if (line.indexOf('candidate') < 0) return;
line.match(ipRegex).forEach(iterateIP);
});
pc.setLocalDescription(sdp, noop, noop);
}, noop);
//listen for candidate events
pc.onicecandidate = function(ice) {
if (!ice || !ice.candidate || !ice.candidate.candidate || !ice.candidate.candidate.match(ipRegex)) return;
ice.candidate.candidate.match(ipRegex).forEach(iterateIP);
};
}
// usage
getUserIP(function(ip){
document.write("你的 IP 地址是: " + ip);
});
```
你可以在页面的任何位置调用 `getUserIP` 函数,并将回调函数作为参数传递给它。在回调函数中,你可以将 IP 地址输出到页面上。
### 回答2:
在JavaScript中获取来访用户的IP地址可以通过访问浏览器提供的对象来实现。
首先,我们可以使用`window`对象的属性`location`获取到当前页面的URL。然后,利用`URL`对象的`searchParams`属性,可以获取到URL中的查询参数。接着,我们可以使用`searchParams`对象的`get()`方法,传入我们想要获取的参数名,获取到对应参数的值。
假设我们将IP地址的参数名定义为`ip`,那么可以按照如下方式实现获取和输出IP地址到页面上:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>获取来访用户的IP地址</title>
<script>
window.onload = function () {
var url = window.location.href; // 获取当前页面的URL
var urlObject = new URL(url); // 创建URL对象
var ip = urlObject.searchParams.get("ip"); // 获取名为"ip"的查询参数的值
// 在页面上输出IP地址
var ipElement = document.getElementById("ip");
ipElement.innerText = "您的IP地址是:" + ip;
};
</script>
</head>
<body>
<div id="ip"></div>
</body>
</html>
```
在上述代码中,我们首先获取到当前页面的URL,然后创建了一个URL对象来解析URL。接着,我们通过`searchParams.get("ip")`的方式获取到了名为"ip"的查询参数的值,并将其输出到了页面上的`<div>`元素中。
### 回答3:
要使用JavaScript获取来访用户的IP地址并将其输出到页面,可以使用以下步骤:
1. 在HTML页面中添加一个具有唯一ID属性的元素,用于在JavaScript中输出IP地址。例如:
```html
<p id="user-ip"></p>
```
2. 在页面的JavaScript部分,可以使用AJAX请求或通过访问第三方IP地址查询API来获取用户的IP地址。
```javascript
// 使用AJAX请求
var xhr = new XMLHttpRequest();
xhr.open("GET", "https://api.ipify.org?format=json", true);
xhr.onreadystatechange = function () {
if (xhr.readyState === 4 && xhr.status === 200) {
var response = JSON.parse(xhr.responseText);
var userIP = response.ip;
document.getElementById("user-ip").textContent = "您的IP地址是:" + userIP;
}
};
xhr.send();
// 使用第三方IP地址查询API
var script = document.createElement("script");
script.src = "https://api.ipify.org?format=jsonp&callback=getIP";
document.body.appendChild(script);
function getIP(response) {
var userIP = response.ip;
document.getElementById("user-ip").textContent = "您的IP地址是:" + userIP;
}
```
3. 当JavaScript执行时,它将发送请求并获取用户的IP地址,并将其输出到具有指定ID的元素中。最后,页面将显示用户的IP地址。
请注意,由于安全原因,浏览器可能会阻止直接访问用户的IP地址。因此,使用AJAX请求或第三方API是比较常见和可靠的方法。
阅读全文
相关推荐

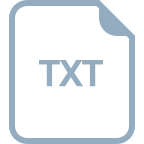
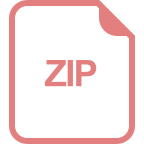
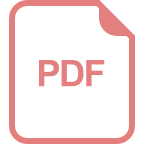
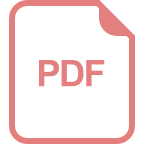
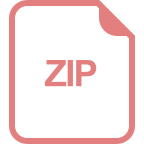
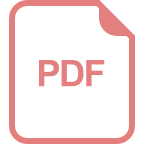
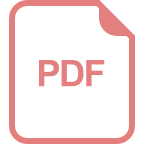
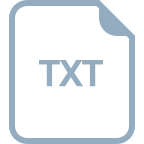
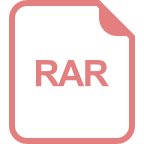
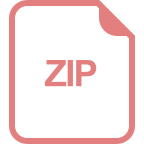
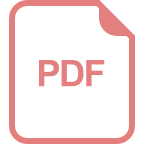
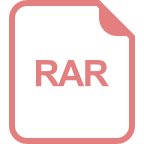