2. 有一个表单,有用户名,密码,年龄及提交选项; 点击提交后, 在下面的表格中产生一条记录; 注意: 1. 初始会有一条表格头信息,只是在后面追加记录 2. 信息头为用户名,密码,年龄,操作 3. 点击删除操作, 可以删除指定记录
时间: 2024-03-10 08:48:11 浏览: 26
这是一个 Web 开发的问题,需要使用 HTML、CSS、JavaScript 和后端语言(如 PHP、Python 等)来实现。下面是一种可能的实现方式:
1. HTML 部分
```html
<form>
<label>用户名:<input type="text" name="username"></label><br>
<label>密码:<input type="password" name="password"></label><br>
<label>年龄:<input type="number" name="age"></label><br>
<button type="button" onclick="addRecord()">提交</button>
</form>
<table>
<thead>
<tr>
<th>用户名</th>
<th>密码</th>
<th>年龄</th>
<th>操作</th>
</tr>
</thead>
<tbody id="recordList">
</tbody>
</table>
```
2. JavaScript 部分
```javascript
// 定义一个数组来保存表格中的记录
let records = [];
// 添加一条记录
function addRecord() {
let form = document.querySelector('form');
let username = form.elements['username'].value;
let password = form.elements['password'].value;
let age = form.elements['age'].value;
records.push({ username, password, age });
renderRecords();
}
// 渲染所有记录
function renderRecords() {
let tbody = document.querySelector('#recordList');
// 清空表格内容
tbody.innerHTML = '';
// 添加表头
let tr = document.createElement('tr');
let th1 = document.createElement('th');
th1.textContent = '用户名';
let th2 = document.createElement('th');
th2.textContent = '密码';
let th3 = document.createElement('th');
th3.textContent = '年龄';
let th4 = document.createElement('th');
th4.textContent = '操作';
tr.appendChild(th1);
tr.appendChild(th2);
tr.appendChild(th3);
tr.appendChild(th4);
tbody.appendChild(tr);
// 添加记录
for (let i = 0; i < records.length; i++) {
let record = records[i];
let tr = document.createElement('tr');
let td1 = document.createElement('td');
td1.textContent = record.username;
let td2 = document.createElement('td');
td2.textContent = record.password;
let td3 = document.createElement('td');
td3.textContent = record.age;
let td4 = document.createElement('td');
let button = document.createElement('button');
button.textContent = '删除';
button.addEventListener('click', () => {
records.splice(i, 1);
renderRecords();
});
td4.appendChild(button);
tr.appendChild(td1);
tr.appendChild(td2);
tr.appendChild(td3);
tr.appendChild(td4);
tbody.appendChild(tr);
}
}
```
3. 后端部分
这里以 PHP 为例,使用文件来保存表格记录。将下面的代码保存为 `submit.php`,并将表单的 `action` 属性设置为该文件的路径。
```php
<?php
// 读取已有记录
$records = json_decode(file_get_contents('records.json'), true) ?? [];
// 添加新记录
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
$username = $_POST['username'] ?? '';
$password = $_POST['password'] ?? '';
$age = $_POST['age'] ?? '';
$records[] = [
'username' => $username,
'password' => $password,
'age' => $age,
];
file_put_contents('records.json', json_encode($records));
}
// 输出所有记录
header('Content-Type: application/json');
echo json_encode($records);
```
上面的代码中,`file_get_contents` 函数用于读取保存记录的 JSON 文件,`json_decode` 函数将其解析为数组。如果文件不存在或解析失败,返回一个空数组。`file_put_contents` 函数用于将记录保存回文件。`header` 函数设置响应头,告诉浏览器返回的是 JSON 格式的数据。`json_encode` 函数将数组转换为 JSON 字符串。
相关推荐
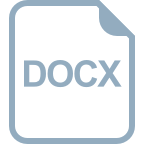
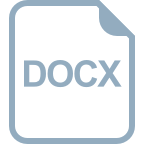
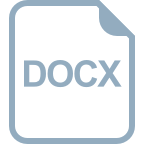














