用java代码多举几个例子
时间: 2024-03-21 16:38:48 浏览: 62
好的,以下是使用Java代码遍历Map并修改其Value的几个例子:
1. 使用entrySet()方法遍历Map
```java
Map<String, Integer> map = new HashMap<>();
map.put("apple", 2);
map.put("banana", 3);
map.put("orange", 4);
for (Map.Entry<String, Integer> entry : map.entrySet()) {
entry.setValue(entry.getValue() + 1);
}
for (Map.Entry<String, Integer> entry : map.entrySet()) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
```
在上述代码中,我们使用`entrySet()`方法遍历Map,并通过`entry.setValue()`方法修改其Value。
2. 使用keySet()方法遍历Map
```java
Map<String, Integer> map = new HashMap<>();
map.put("apple", 2);
map.put("banana", 3);
map.put("orange", 4);
for (String key : map.keySet()) {
map.put(key, map.get(key) + 1);
}
for (Map.Entry<String, Integer> entry : map.entrySet()) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
```
在上述代码中,我们使用`keySet()`方法遍历Map,并通过`map.put(key, map.get(key) + 1)`方法修改其Value。
3. 使用forEach()方法遍历Map
```java
Map<String, Integer> map = new HashMap<>();
map.put("apple", 2);
map.put("banana", 3);
map.put("orange", 4);
map.forEach((key, value) -> map.put(key, value + 1));
map.forEach((key, value) -> System.out.println(key + ": " + value));
```
在上述代码中,我们使用`forEach()`方法遍历Map,并通过Lambda表达式修改其Value。需要注意的是,我们在Lambda表达式中使用`map.put(key, value + 1)`方法修改Value,而不是直接使用`value += 1`,因为Lambda表达式中的变量必须是final或effectively final的。
阅读全文
相关推荐



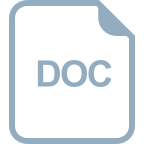

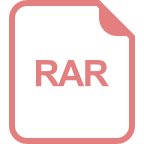
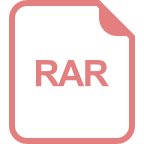
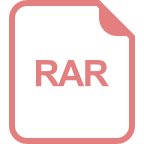





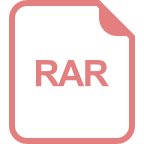

