python用0 12 34 剪刀石头布
时间: 2023-12-22 08:04:10 浏览: 24
以下是一个简单的示例程序,其中使用了random模块来随机生成计算机的选择,通过input函数来获取玩家的选择,并使用if-else语句来判断胜负。
```python
import random
print("欢迎来玩剪刀石头布!")
print("请出拳:0-剪刀,1-石头,2-布")
# 生成计算机的选择
computer = random.randint(0, 2)
# 获取玩家的选择
player = int(input("请出拳:"))
# 判断胜负
if player == computer:
print("平局")
elif player == 0 and computer == 1:
print("你输了")
elif player == 0 and computer == 2:
print("你赢了")
elif player == 1 and computer == 0:
print("你赢了")
elif player == 1 and computer == 2:
print("你输了")
elif player == 2 and computer == 0:
print("你输了")
elif player == 2 and computer == 1:
print("你赢了")
else:
print("无效的选择")
```
注意:此示例程序仅供学习参考,实际应用中可能需要进行更多的判断和优化。
相关问题
python编程设计一个剪刀石头布游戏
在Python中设计一个简单的剪刀、石头、布游戏,可以遵循以下步骤:
1. **创建玩家选择函数**:
```python
def player_choice():
choices = ["石头", "剪刀", "布"]
return input("请输入你的选择(石头、剪刀、布):").lower().strip() in choices
```
2. **创建电脑选择函数**:
```python
import random
def computer_choice():
return random.choice(["石头", "剪刀", "布"])
```
3. **游戏规则判断函数**:
```python
def compare_choices(player, computer):
if player == computer:
return "平局"
elif (player == "石头" and computer == "剪刀") or \
(player == "剪刀" and computer == "布") or \
(player == "布" and computer == "石头"):
return "你赢了!"
else:
return "电脑赢了!"
```
4. **主循环**:
```python
while True:
player_win = False
player_choice_str = player_choice()
print(f"你选择了 {player_choice_str},电脑选择...")
computer_choice_str = computer_choice()
result = compare_choices(player_choice_str, computer_choice_str)
print(result)
if "你赢了!" in result or "电脑赢了!" in result:
player_win = True
break
if player_win:
print("恭喜你赢得了这场比赛!")
else:
print("很遗憾,你输了。")
```
5. **结束游戏提示**:
添加一些游戏结束的条件,如用户请求退出,或者达到一定的胜利次数等。
完整代码示例:
```python
def main():
# ... (以上所有代码段)
if __name__ == "__main__":
main()
```
python 猜丁壳完成剪刀石头布游戏代码
好的,以下是 Python 猜拳游戏代码:
```python
import random
print("欢迎来到猜拳游戏!")
while True:
# 用户出拳
user_choice = input("请出拳(剪刀/石头/布):")
while user_choice not in ["剪刀", "石头", "布"]:
user_choice = input("输入有误,请重新出拳(剪刀/石头/布):")
# 电脑出拳
computer_choice = random.choice(["剪刀", "石头", "布"])
print("电脑出拳:", computer_choice)
# 判断胜负
if user_choice == computer_choice:
print("平局!")
elif (user_choice == "剪刀" and computer_choice == "布") or \
(user_choice == "石头" and computer_choice == "剪刀") or \
(user_choice == "布" and computer_choice == "石头"):
print("恭喜你,你赢了!")
else:
print("很遗憾,你输了!")
# 询问是否继续
choice = input("是否继续(是/否):")
while choice not in ["是", "否"]:
choice = input("输入有误,请重新选择(是/否):")
if choice == "否":
break
print("游戏结束,谢谢参与!")
```
使用方法:运行程序后,按照提示输入剪刀、石头或布,程序会自动随机出拳并判断胜负。最后询问是否继续,输入“是”则继续,输入“否”则结束游戏。
阅读全文
相关推荐
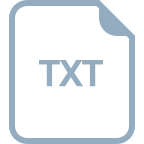
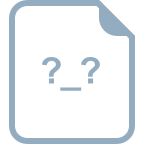
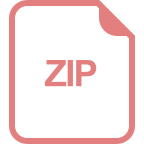
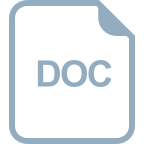
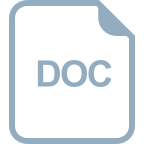
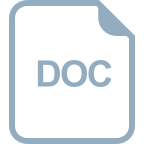







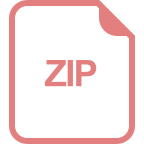
