设计一个数字输入框组件,只能输入数字,附带两个按钮用于加1和减1。也可以自行添加初始值、最大值、最小值的按钮。在输入框中的数值改变时,触发一个自定义事件来通知父组件。(vue)
时间: 2024-02-22 19:57:29 浏览: 200
好的,根据您的需求,可以参考下面的代码实现:
```html
<template>
<div>
<input type="number" v-model.number="value" @input="handleChange">
<button @click="handleAdd">+1</button>
<button @click="handleMinus">-1</button>
<button @click="handleInit">初始值</button>
<button @click="handleMin">最小值</button>
<button @click="handleMax">最大值</button>
</div>
</template>
<script>
export default {
props: {
value: {
type: Number,
default: 0
},
min: {
type: Number,
default: Number.MIN_SAFE_INTEGER
},
max: {
type: Number,
default: Number.MAX_SAFE_INTEGER
},
init: {
type: Number,
default: 0
}
},
methods: {
handleChange() {
this.$emit('change', this.value);
},
handleAdd() {
if (this.value + 1 > this.max) {
this.value = this.max;
} else {
this.value += 1;
}
this.$emit('change', this.value);
},
handleMinus() {
if (this.value - 1 < this.min) {
this.value = this.min;
} else {
this.value -= 1;
}
this.$emit('change', this.value);
},
handleInit() {
this.value = this.init;
this.$emit('change', this.value);
},
handleMin() {
this.value = this.min;
this.$emit('change', this.value);
},
handleMax() {
this.value = this.max;
this.$emit('change', this.value);
}
}
}
</script>
```
这个组件有以下几个属性:
- `value`:输入框中的数值,需要使用 `.number` 修饰符将其转换为数字类型。
- `min`:最小值,默认值是 `Number.MIN_SAFE_INTEGER`。
- `max`:最大值,默认值是 `Number.MAX_SAFE_INTEGER`。
- `init`:初始值,默认值是 `0`。
在 `methods` 中,我们定义了以下几个方法:
- `handleChange()`:当输入框中的数值变化时,触发该方法,并使用 `$emit` 方法向父组件发送 `change` 事件,同时将当前数值作为参数传递。
- `handleAdd()`:加1按钮的点击事件,将当前数值加1,并判断是否超出最大值,如果超出则将数值设置为最大值。
- `handleMinus()`:减1按钮的点击事件,将当前数值减1,并判断是否超出最小值,如果超出则将数值设置为最小值。
- `handleInit()`:初始值按钮的点击事件,将数值设置为初始值。
- `handleMin()`:最小值按钮的点击事件,将数值设置为最小值。
- `handleMax()`:最大值按钮的点击事件,将数值设置为最大值。
在模板中,我们使用 `v-model.number` 将 `value` 和输入框绑定,同时监听 `@input` 事件,触发 `handleChange()` 方法。另外,我们还定义了五个按钮,并分别绑定对应的点击事件。
在父组件中,可以通过监听 `change` 事件获取输入框中的数值,并进行相应的处理。例如:
```html
<template>
<div>
<number-input :value="count" @change="handleChange"></number-input>
<p>当前数量:{{ count }}</p>
</div>
</template>
<script>
import NumberInput from './NumberInput.vue';
export default {
components: {
NumberInput
},
data() {
return {
count: 0
};
},
methods: {
handleChange(value) {
this.count = value;
}
}
}
</script>
```
在这个父组件中,我们使用了 `NumberInput` 组件,并将 `count` 绑定到 `value` 属性上。当 `NumberInput` 组件中的数值变化时,会触发 `change` 事件,我们可以在父组件中监听该事件,并将数值赋值给 `count`,从而实现父子组件之间的通信。
阅读全文
相关推荐
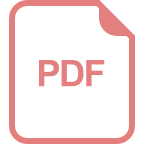
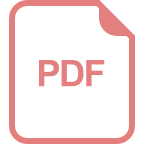
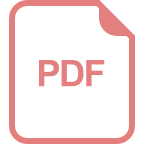














