vue+element ui实现评论功能
时间: 2023-05-28 14:01:09 浏览: 887
在Vue Element UI中实现评论功能需要依赖一些组件,如表单、输入框、按钮、弹窗等。下面是一个简单的评论功能实现步骤:
1. 引入Element UI组件库
在Vue项目中,可以通过npm安装Element UI组件库,并在main.js文件中引入。
```
npm install element-ui --save
import Vue from 'vue';
import ElementUI from 'element-ui';
import 'element-ui/lib/theme-chalk/index.css';
Vue.use(ElementUI);
```
2. 创建评论组件
在Vue组件中,可以创建一个评论组件,用于显示评论列表和评论表单。在该组件中,需要定义data属性,用于存储评论列表和表单数据。
```
<template>
<div>
<div v-for="comment in comments" :key="comment.id">{{comment.content}}</div>
<el-form :model="form" label-position="left" label-width="80px" inline>
<el-form-item label="评论">
<el-input v-model="form.content" placeholder="请输入评论内容"></el-input>
</el-form-item>
<el-form-item>
<el-button type="primary" @click="addComment">发表评论</el-button>
</el-form-item>
</el-form>
</div>
</template>
<script>
export default {
data() {
return {
comments: [],
form: {
content: ''
}
}
},
methods: {
addComment() {
const comment = {
id: Date.now(),
content: this.form.content
}
this.comments.push(comment)
this.form.content = ''
}
}
}
</script>
```
在上面的代码中,el-form表示表单,el-input表示输入框,el-button表示按钮。comments数组用于存储评论列表,form对象用于存储表单数据。addComment方法用于发表评论,将表单数据存储到comments数组中,并清空表单数据。
3. 在父组件中使用评论组件
在父组件中使用评论组件,并将评论列表传递给该组件。
```
<template>
<div>
<comment :comments="comments"></comment>
</div>
</template>
<script>
import Comment from './Comment.vue'
export default {
data() {
return {
comments: [
{id: 1, content: '这是一条评论'},
{id: 2, content: '这是另一条评论'}
]
}
},
components: {
Comment
}
}
</script>
```
在上面的代码中,comment组件通过属性comments获取评论列表,并在父组件中定义comments数组,存储评论数据。
4. 给评论组件添加弹窗
在评论组件中,可以添加一个弹窗组件,用于提示评论成功。
```
<template>
<div>
<div v-for="comment in comments" :key="comment.id">{{comment.content}}</div>
<el-form :model="form" label-position="left" label-width="80px" inline>
<el-form-item label="评论">
<el-input v-model="form.content" placeholder="请输入评论内容"></el-input>
</el-form-item>
<el-form-item>
<el-button type="primary" @click="addComment">发表评论</el-button>
</el-form-item>
</el-form>
<el-dialog title="提示" :visible.sync="dialogVisible">
<p>评论成功!</p>
</el-dialog>
</div>
</template>
<script>
export default {
data() {
return {
comments: [],
form: {
content: ''
},
dialogVisible: false
}
},
methods: {
addComment() {
const comment = {
id: Date.now(),
content: this.form.content
}
this.comments.push(comment)
this.form.content = ''
this.dialogVisible = true
setTimeout(() => {
this.dialogVisible = false
}, 2000)
}
}
}
</script>
```
在上面的代码中,el-dialog表示弹窗,dialogVisible属性用于控制弹窗的显示/隐藏。在addComment方法中,添加评论成功后,弹出评论成功的提示,并在2秒后关闭弹窗。
这就是一个简单的Vue Element UI评论功能实现。
相关推荐
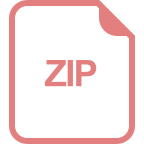













