c++实现一维数组与指针,题目如下,键盘输入若干正整数到一个数组中(个数不定,以-1结束,超过2000个数据则只取前2000个),将其调整为所有奇数在前,偶数在后。要求建立三个子函数通过调用的方式来完成
时间: 2023-10-04 13:11:24 浏览: 56
好的,下面是具体的实现。
首先,我们需要创建一个一维数组,并从键盘读入若干个正整数。在读入的过程中,我们需要判断是否已经读入了超过2000个数据或者读入了-1,如果是则退出读入。代码如下:
```c++
#include <iostream>
using namespace std;
const int MAXSIZE = 2000;
void input(int* arr, int& n) {
cout << "请输入若干正整数,以-1结束:" << endl;
n = 0;
while (n < MAXSIZE) {
int x;
cin >> x;
if (x == -1) break;
arr[n++] = x;
}
}
```
接下来,我们需要将数组中的元素按照奇数和偶数分别放到数组的前面和后面。我们可以采用双指针的方式,一个指针从数组的前面开始扫描,另一个指针从数组的后面开始扫描,当两个指针相遇时,排序完成。具体的实现代码如下:
```c++
void adjust(int* arr, int n) {
int i = 0, j = n - 1;
while (i < j) {
while (i < j && arr[i] % 2 == 1) i++;
while (i < j && arr[j] % 2 == 0) j--;
if (i < j) swap(arr[i++], arr[j--]);
}
}
```
最后,我们需要输出排序后的数组。代码如下:
```c++
void output(int* arr, int n) {
cout << "排序后的数组为:" << endl;
for (int i = 0; i < n; i++) {
cout << arr[i] << " ";
}
cout << endl;
}
```
将上述三个函数组合起来,完整的代码如下:
```c++
#include <iostream>
using namespace std;
const int MAXSIZE = 2000;
void input(int* arr, int& n) {
cout << "请输入若干正整数,以-1结束:" << endl;
n = 0;
while (n < MAXSIZE) {
int x;
cin >> x;
if (x == -1) break;
arr[n++] = x;
}
}
void adjust(int* arr, int n) {
int i = 0, j = n - 1;
while (i < j) {
while (i < j && arr[i] % 2 == 1) i++;
while (i < j && arr[j] % 2 == 0) j--;
if (i < j) swap(arr[i++], arr[j--]);
}
}
void output(int* arr, int n) {
cout << "排序后的数组为:" << endl;
for (int i = 0; i < n; i++) {
cout << arr[i] << " ";
}
cout << endl;
}
int main() {
int arr[MAXSIZE];
int n;
input(arr, n);
adjust(arr, n);
output(arr, n);
return 0;
}
```
希望对你有所帮助!
相关推荐
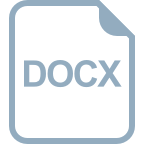
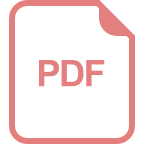
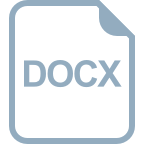














