两个进程使用poxis信号量同步
时间: 2024-01-24 17:04:30 浏览: 24
使用 POSIX 信号量进行进程间同步可以分为以下几个步骤:
1. 创建信号量:使用 `sem_open` 函数创建一个新的信号量或打开一个已经存在的信号量。
```c
#include <semaphore.h>
sem_t *sem_open(const char *name, int oflag, mode_t mode, unsigned int value);
```
其中,`name` 参数为信号量的名称,`oflag` 参数指定打开或创建标志,`mode` 参数指定权限位,`value` 参数指定信号量的初始值。若成功返回一个指向信号量对象的指针,否则返回 `SEM_FAILED`。
2. 进程等待信号量:使用 `sem_wait` 函数等待信号量。若信号量的值大于 0,`sem_wait` 函数会将信号量值减 1 并立即返回;否则,它会阻塞进程直到信号量可用。
```c
#include <semaphore.h>
int sem_wait(sem_t *sem);
```
其中,`sem` 参数为指向信号量对象的指针。若成功返回 0,否则返回 -1。
3. 进程释放信号量:使用 `sem_post` 函数释放信号量。`sem_post` 函数将信号量值加 1,并唤醒一个等待的进程。
```c
#include <semaphore.h>
int sem_post(sem_t *sem);
```
其中,`sem` 参数为指向信号量对象的指针。若成功返回 0,否则返回 -1。
4. 关闭信号量:使用 `sem_close` 函数关闭一个打开的信号量。
```c
#include <semaphore.h>
int sem_close(sem_t *sem);
```
其中,`sem` 参数为指向信号量对象的指针。若成功返回 0,否则返回 -1。
下面是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <semaphore.h>
#include <fcntl.h>
#include <unistd.h>
int main()
{
sem_t *sem = sem_open("my_semaphore", O_CREAT, 0644, 1);
if (sem == SEM_FAILED) {
perror("sem_open");
exit(EXIT_FAILURE);
}
pid_t pid = fork();
if (pid < 0) {
perror("fork");
exit(EXIT_FAILURE);
}
else if (pid == 0) {
// 子进程
sem_wait(sem);
printf("Child process: got the semaphore\n");
sem_post(sem);
exit(EXIT_SUCCESS);
}
else {
// 父进程
sem_wait(sem);
printf("Parent process: got the semaphore\n");
sem_post(sem);
wait(NULL);
sem_close(sem);
sem_unlink("my_semaphore");
exit(EXIT_SUCCESS);
}
}
```
在这个示例中,我们创建了一个名为 `my_semaphore` 的信号量,并将其初始值设置为 1。然后创建了一个子进程,父进程和子进程都调用了 `sem_wait` 函数等待信号量。
由于初始值为 1,因此父进程可以先获取到信号量,输出 "Parent process: got the semaphore",然后释放信号量并等待子进程结束。子进程在父进程释放信号量后获取信号量,输出 "Child process: got the semaphore",然后释放信号量并退出。最后父进程关闭信号量并删除对应的信号量对象。
相关推荐
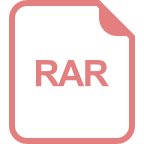
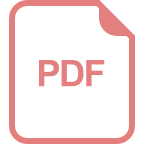














