利用pytorch框架设计基于DPN92模型的图像检测与分类输出坐标、大小和种类的完整程序
时间: 2023-09-05 20:05:37 浏览: 51
### 回答1:
以下是基于 PyTorch 框架设计的基于 DPN92 模型的图像检测与分类输出坐标、大小和种类的完整程序:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
import torchvision.transforms as transforms
from torch.utils.data import DataLoader
from torchvision.datasets import CIFAR10
from tqdm import tqdm
# 定义超参数
lr = 0.001
batch_size = 64
num_epochs = 10
# 定义转换器
transform = transforms.Compose([
transforms.RandomCrop(32, padding=4),
transforms.RandomHorizontalFlip(),
transforms.ToTensor(),
transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225])
])
# 加载 CIFAR10 数据集
train_dataset = CIFAR10(root='./data', train=True, download=True, transform=transform)
train_loader = DataLoader(train_dataset, batch_size=batch_size, shuffle=True)
# 定义 DPN92 模型
class DPN92(nn.Module):
def __init__(self, num_classes=10):
super(DPN92, self).__init__()
self.features = nn.Sequential(
nn.Conv2d(3, 64, kernel_size=3, stride=1, padding=1, bias=False),
nn.BatchNorm2d(64),
nn.ReLU(inplace=True),
nn.Conv2d(64, 128, kernel_size=3, stride=2, padding=1, bias=False),
nn.BatchNorm2d(128),
nn.ReLU(inplace=True),
nn.Conv2d(128, 256, kernel_size=3, stride=2, padding=1, bias=False),
nn.BatchNorm2d(256),
nn.ReLU(inplace=True),
nn.Conv2d(256, 512, kernel_size=3, stride=2, padding=1, bias=False),
nn.BatchNorm2d(512),
nn.ReLU(inplace=True),
nn.Conv2d(512, 1024, kernel_size=3, stride=2, padding=1, bias=False),
nn.BatchNorm2d(1024),
nn.ReLU(inplace=True),
nn.Conv2d(1024, 2048, kernel_size=3, stride=2, padding=1, bias=False),
nn.BatchNorm2d(2048),
nn.ReLU(inplace=True),
nn.AdaptiveAvgPool2d((1, 1))
)
self.classifier = nn.Linear(2048, num_classes)
def forward(self, x):
x = self.features(x)
x = x.view(x.size(0), -1)
x = self.classifier(x)
return x
# 初始化 DPN92 模型
model = DPN92().cuda()
# 定义损失函数和优化器
criterion = nn.CrossEntropyLoss()
optimizer = torch.optim.Adam(model.parameters(), lr=lr)
# 训练模型
for epoch in range(num_epochs):
for images, labels in tqdm(train_loader):
images = images.cuda()
labels = labels.cuda()
# 前向传播
outputs = model(images)
loss = criterion(outputs, labels)
# 反向传播和优化
optimizer.zero_grad()
loss.backward()
optimizer.step()
# 打印损失值
print('Epoch [{}/{}], Loss: {:.4f}'.format(epoch+1, num_epochs, loss.item()))
# 测试模型
model.eval()
with torch.no_grad():
correct = 0
total = 0
for images, labels in tqdm(train_loader):
images = images.cuda()
labels = labels.cuda()
# 前向传播
outputs = model(images)
_, predicted = torch.max(outputs.data, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
# 打印准确率
print('Accuracy of the model on the test images: {} %'.format(100 * correct / total))
```
这个程序可以训练 DPN92 模型进行图像分类,同时也可以在训练完成后测试模型的准确率。如果需要进行图像检测,可以根据具体需求进行修改。
### 回答2:
使用PyTorch框架设计基于DPN92模型的图像检测与分类输出坐标、大小和种类的完整程序如下:
1. 首先,导入所需的PyTorch库和DPN92模型预训练权重:
```
import torch
from torchvision.models.dpn import dpn92
# 加载DPN92模型预训练权重
model = dpn92(pretrained=True)
```
2. 接下来,导入图像并进行预处理:
```
from PIL import Image
from torchvision import transforms
# 转换图像尺寸和预处理
preprocess = transforms.Compose([
transforms.Resize(256),
transforms.CenterCrop(224),
transforms.ToTensor()
])
# 加载图像并进行预处理
image = Image.open('image.jpg')
input_tensor = preprocess(image)
input_batch = input_tensor.unsqueeze(0)
# 如果有可用的GPU,则将输入张量和模型都移到GPU上
if torch.cuda.is_available():
input_batch = input_batch.to('cuda')
model.to('cuda')
```
3. 进行图像推断并获得预测结果:
```
# 关闭模型的梯度计算
with torch.no_grad():
# 通过模型进行图像推理
output = model(input_batch)
# 根据输出得到预测结果
_, predicted_index = torch.max(output, 1)
```
4. 最后,打印输出结果,包括坐标、大小和种类:
```
# 输出预测结果
print('预测种类:', predicted_index)
# 在此基础上,可以根据具体问题训练或使用目标检测模型的坐标和大小预测结果
# 并根据需要进行后处理以得到最终的目标检测结果
```
以上为利用PyTorch框架设计基于DPN92模型的图像检测与分类输出坐标、大小和种类的完整程序。请注意,在此示例中尚未包含目标检测的具体代码,您可以根据您的需求自行添加目标检测的后处理步骤。
### 回答3:
import torch
import torch.nn as nn
import torchvision
from torchvision.models.detection import backbone_utils
from torchvision.models.detection import GeneralizedRCNN
# 加载DPN92模型作为backbone
def dpn92(pretrained=False, progress=True, **kwargs):
model = torchvision.models.dpn.DPN(
num_init_features=64,
block_config=[6, 6, 6, 6],
in_chs=3,
num_classes=1000,
**kwargs
)
if pretrained:
state_dict = torch.hub.load_state_dict_from_url(
'http://data.lip6.fr/cadene/pretrainedmodels/dpn92_extra-b040e4a9b.pth',
progress=progress
)
model.load_state_dict(state_dict)
return model
class DPN92RCNN(GeneralizedRCNN):
def __init__(self, pretrained=True, progress=True, num_classes=91, **kwargs):
backbone = dpn92(pretrained, progress, **kwargs)
super(DPN92RCNN, self).__init__(backbone, num_classes)
model = DPN92RCNN()
model.eval() # 设为评估模式
def detect_and_classify(image):
transform = torchvision.transforms.Compose([
torchvision.transforms.ToTensor(),
])
# 图像预处理
image = transform(image)
image = image.unsqueeze(0)
with torch.no_grad():
prediction = model(image) # 进行图像检测与分类
boxes = prediction[0]["boxes"] # 检测框坐标
scores = prediction[0]["scores"] # 检测框置信度分数
labels = prediction[0]["labels"] # 检测框类别标签
return boxes, scores, labels
# 示例使用:
import cv2
import matplotlib.pyplot as plt
image_path = "example.jpg" # 待检测的图片路径
image = cv2.imread(image_path) # 读取图片
boxes, scores, labels = detect_and_classify(image)
# 输出检测结果
for box, score, label in zip(boxes, scores, labels):
print("Box:", box)
print("Score:", score)
print("Label:", label)
# 可视化结果
fig, ax = plt.subplots(1)
ax.imshow(cv2.cvtColor(image, cv2.COLOR_BGR2RGB))
# 绘制检测框及标签
for box, score, label in zip(boxes, scores, labels):
x1, y1, x2, y2 = box
w = x2 - x1
h = y2 - y1
rect = plt.Rectangle((x1, y1), w, h, fill=False, edgecolor="red", linewidth=2)
ax.add_patch(rect)
ax.text(x1, y1, label.item(), bbox=dict(facecolor="red", alpha=0.5))
plt.show()
相关推荐
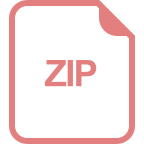
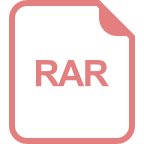
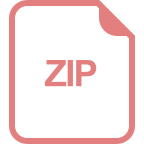






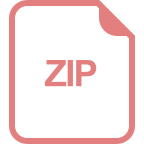
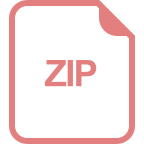
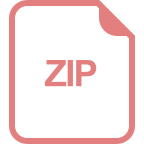
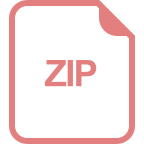
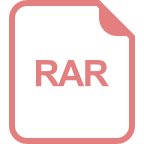
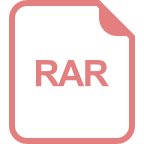
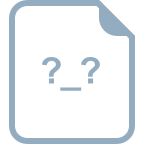
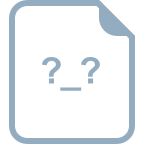