ICCP算法是一种用于重力匹配导航的算法,使用ICCP算法需要先找到航迹的重力等值线,如何找到等值线
时间: 2023-06-11 08:08:01 浏览: 243
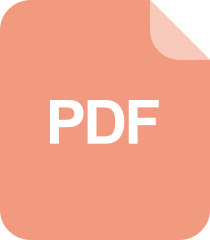
一种导航地图匹配算法
要找到航迹的重力等值线,可以使用以下步骤:
1. 首先,需要获取航迹的高度数据。可以使用卫星高度测量仪、雷达高度测量仪等工具获取高度数据。
2. 接着,可以使用插值算法将高度数据转化为连续的高度曲面。
3. 然后,可以使用等值线提取算法,如Marching Squares算法、Contour Tree算法等,从高度曲面中提取出等高线。
4. 最后,可以根据等高线的密度和间隔,选择合适的等高线作为航迹的重力等值线。
需要注意的是,不同的等值线提取算法具有不同的适用范围和优缺点,需要根据具体的数据特点和应用场景选择合适的算法。
阅读全文
相关推荐
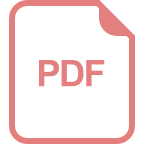

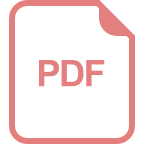
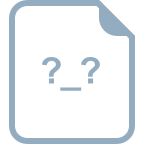
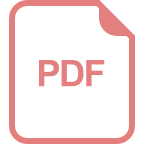
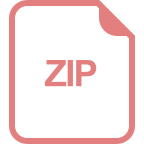
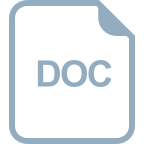
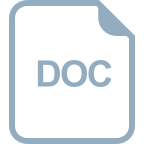
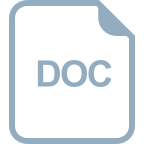
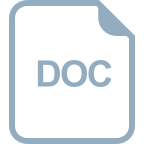
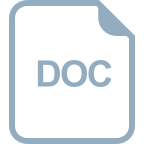