c#怎么下载使用iTextSharp
时间: 2023-12-30 10:02:16 浏览: 61
在 C# 中使用 iTextSharp 库需要先添加 iTextSharp 的引用。可以通过 NuGet 包管理器或手动下载并添加到项目中。
以下是通过 NuGet 包管理器添加 iTextSharp 的方法:
1. 打开 Visual Studio,打开要使用 iTextSharp 的项目。
2. 在项目中右键点击“引用”并选择“管理 NuGet 程序包”。
3. 在“NuGet 程序包管理器”中搜索“iTextSharp”,选择并安装它。
4. 安装完成后,即可在代码文件中使用 `using iTextSharp.text;` 和 `using iTextSharp.text.pdf;` 引用 iTextSharp 中的类和方法。
如果需要手动下载 iTextSharp,可以按照以下步骤进行:
1. 打开 iTextSharp 的官网(https://itextpdf.com/en/products/itextsharp)。
2. 点击“Download”进入下载页面。
3. 选择适合自己的版本(一般选择最新版本)并下载。
4. 将 iTextSharp 的 dll 文件添加到项目中,并在代码文件中使用 `using iTextSharp.text;` 和 `using iTextSharp.text.pdf;` 引用 iTextSharp 中的类和方法。
无论是通过 NuGet 包管理器还是手动下载,添加引用后即可在代码文件中使用 iTextSharp。
相关问题
C# iTextSharp
C# iTextSharp是一个用于处理PDF文件的开源库。它可以实现多种功能,比如添加水印、下载字节流、设置文件为只读、拆分PDF和合并PDF等。
使用C# iTextSharp库可以很方便地实现这些功能。例如,要添加水印,可以使用该库提供的API来在PDF文件上添加文本或图像水印。要下载字节流到本地,可以使用库中的函数将PDF文件转换为字节数组,并将字节数组写入文件流保存到本地。要将文档设置为只读,可以使用该库提供的功能来设置PDF文件的属性,将其设置为只读模式。要拆分PDF,可以使用库中的方法按照页面或书签进行拆分。要合并PDF,可以使用该库提供的函数将多个PDF文件合并为一个。
请注意,上述提到的版本号5.5.12.0是iTextSharp的一个特定版本,具体的使用方式可能会有所不同,因此建议查阅相关文档或参考示例代码以获取更详细的使用方法。<span class="em">1</span>
C# itextsharp
C# iTextSharp是一个用于增强与PDF功能的应用程序的开发人员的Java,.NET,Android和GAE库。iTextSharp是iText的.NET端口,它允许开发人员在.NET平台上使用iText的功能。iTextSharp可以用于创建PDF文档,添加内容,修改现有文档以及从PDF文档中提取文本和图像等。如果需要更深入地了解iTextSharp组件的相关信息,可以查看API文档和iTextSharp产品介绍。在.NET中实现PDF打印的组件比较多,iTextSharp是其中之一。
相关推荐
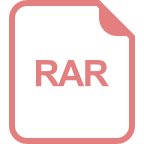
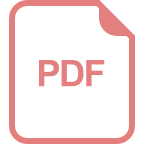
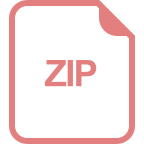












