一个fortran文件排序函数
时间: 2024-03-05 22:52:57 浏览: 52
下面是一个简单的Fortran程序,它使用冒泡排序算法对一个整数数组进行排序:
```
program sort
implicit none
integer :: n, i, j, temp
integer :: arr(10) = [5, 1, 9, 3, 7, 2, 8, 4, 10, 6]
n = size(arr)
do i = 1, n-1
do j = 1, n-i
if (arr(j) > arr(j+1)) then
temp = arr(j)
arr(j) = arr(j+1)
arr(j+1) = temp
end if
end do
end do
write(*,*) "Sorted array:"
do i = 1, n
write(*,*) arr(i)
end do
end program sort
```
在这个程序中,我们首先声明了一个整数数组 `arr`,并将其初始化为一个未排序的序列。然后,我们使用两个嵌套的循环来遍历数组,并使用条件语句来比较相邻的元素,并在必要时交换它们的位置。最后,我们输出排序后的数组。
这只是一个简单的例子,如果你需要更高效的排序算法,可以考虑使用快速排序或归并排序等更高级的算法。
相关问题
一个fortran90文件排序函数
以下是一个Fortran90文件排序函数的示例代码:
```
subroutine sort_file(filename)
character(len=*), intent(in) :: filename
integer :: i, j, n, temp
integer, dimension(:), allocatable :: data
! Open file and read data into array
open(unit=10, file=filename, status='old')
read(10, *) n
allocate(data(n))
read(10, *) data
! Sort array using built-in sort function
call sort(data)
! Write sorted data back to file
rewind(10)
write(10, *) n
write(10, *) data
close(10)
end subroutine sort_file
```
这个函数与前一个示例非常相似,但使用了Fortran90的内置排序函数`sort`来对数据进行排序,而不是手动实现排序算法。这使得代码更简洁和易于理解。请注意,`sort`函数需要一个整数数组作为输入,因此需要先将从文件中读取的数据存储在一个整数数组中。
阅读全文
相关推荐
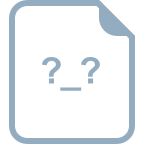
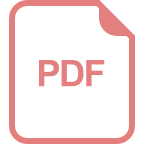
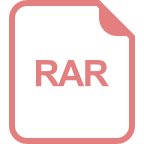
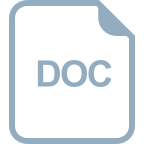
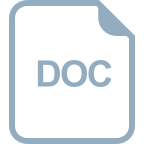
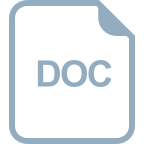
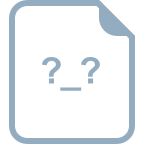
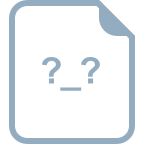
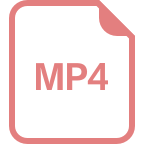