用C语言调用进程函数写一个进程通讯的源文件
时间: 2023-03-23 14:03:58 浏览: 69
首先,需要在C语言中包含进程通讯相关的头文件,如<sys/types.h>、<sys/ipc.h>、<sys/msg.h>等。
然后,可以定义一个消息结构体,用来存储进程间通讯的消息内容。例如:
```
struct message {
long mtype; // 消息类型
char mtext[256]; // 消息内容
};
```
接下来,可以使用msgget()函数创建一个消息队列,用来存储进程间通讯的消息。例如:
```
key_t key = ftok(".", 'a'); // 生成消息队列的key值
int msgid = msgget(key, IPC_CREAT | 0666); // 创建消息队列
```
然后,可以使用fork()函数创建一个子进程,用来发送和接收消息。例如:
```
pid_t pid = fork();
if (pid == ) { // 子进程
// 发送消息
struct message msg;
msg.mtype = 1;
strcpy(msg.mtext, "Hello, parent process!");
msgsnd(msgid, &msg, sizeof(msg.mtext), );
// 接收消息
msgrcv(msgid, &msg, sizeof(msg.mtext), 2, );
printf("Child process received message: %s\n", msg.mtext);
} else if (pid > ) { // 父进程
// 接收消息
struct message msg;
msgrcv(msgid, &msg, sizeof(msg.mtext), 1, );
printf("Parent process received message: %s\n", msg.mtext);
// 发送消息
msg.mtype = 2;
strcpy(msg.mtext, "Hello, child process!");
msgsnd(msgid, &msg, sizeof(msg.mtext), );
} else { // 创建子进程失败
perror("fork");
exit(1);
}
```
最后,需要使用msgctl()函数删除消息队列,释放资源。例如:
```
msgctl(msgid, IPC_RMID, NULL);
```
这样,一个简单的进程通讯的源文件就完成了。完整的代码如下:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
struct message {
long mtype;
char mtext[256];
};
int main() {
key_t key = ftok(".", 'a');
int msgid = msgget(key, IPC_CREAT | 0666);
pid_t pid = fork();
if (pid == ) { // 子进程
// 发送消息
struct message msg;
msg.mtype = 1;
strcpy(msg.mtext, "Hello, parent process!");
msgsnd(msgid, &msg, sizeof(msg.mtext), );
// 接收消息
msgrcv(msgid, &msg, sizeof(msg.mtext), 2, );
printf("Child process received message: %s\n", msg.mtext);
} else if (pid > ) { // 父进程
// 接收消息
struct message msg;
msgrcv(msgid, &msg, sizeof(msg.mtext), 1, );
printf("Parent process received message: %s\n", msg.mtext);
// 发送消息
msg.mtype = 2;
strcpy(msg.mtext, "Hello, child process!");
msgsnd(msgid, &msg, sizeof(msg.mtext), );
} else { // 创建子进程失败
perror("fork");
exit(1);
}
msgctl(msgid, IPC_RMID, NULL);
return ;
}
```
相关推荐
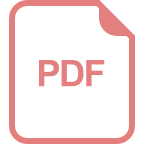
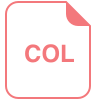
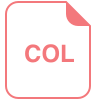
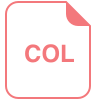
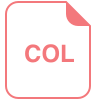
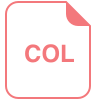









