自定义一个模块,然后在其他源文件中进行调用、测试。
时间: 2024-02-03 14:12:22 浏览: 141
好的,以下是一个简单的示例:
我们可以自定义一个名为 `my_module.py` 的模块,其中包含一个用于计算两个数之和的函数 `add_numbers()` 和一个用于输出一条简单信息的函数 `say_hello()`:
```python
def add_numbers(a, b):
return a + b
def say_hello():
print("Hello, world!")
```
然后,在另一个源文件中,我们可以通过 `import` 语句引入该模块,并使用其中的函数:
```python
import my_module
# 调用 add_numbers() 函数
result = my_module.add_numbers(3, 4)
print(result)
# 调用 say_hello() 函数
my_module.say_hello()
```
输出将会是:
```
7
Hello, world!
```
这样就完成了在其他源文件中调用和测试自定义模块的过程。
相关问题
自定义一个模块,然后在其他源文件中进行调用、测试
好的,下面是一个自定义模块的示例,其中包含一个函数,可以将一个列表中所有元素都平方后返回:
```python
# custom_module.py
def square_list(lst):
"""
将列表中的每个元素平方后返回
"""
return [x ** 2 for x in lst]
```
接下来,我们可以在另一个源文件中导入并使用这个自定义模块:
```python
# main.py
import custom_module
lst = [1, 2, 3, 4, 5]
squared_lst = custom_module.square_list(lst)
print(squared_lst)
```
这段代码导入了我们刚刚定义的 `custom_module` 模块,并使用 `square_list` 函数将列表中的元素平方后返回,并将结果打印出来。运行 `main.py`,可以得到以下输出:
```
[1, 4, 9, 16, 25]
```
这说明我们自定义的模块和函数已经成功地被导入和调用了。
.自定义一个模块,然后在其他源文件中进行调用、测试
好的,我来给你一个简单的示例。
首先,我们创建一个名为 `my_module.py` 的 Python 模块,代码如下:
```python
def multiply_numbers(a, b):
"""
Multiply two numbers and return the result
"""
return a * b
```
这个模块包含了一个名为 `multiply_numbers` 的函数,它可以对两个数字进行乘法运算并返回结果。
接下来,我们可以在其他 Python 源文件中调用这个模块。例如,我们创建一个名为 `test.py` 的文件,代码如下:
```python
import my_module
result = my_module.multiply_numbers(2, 3)
print(result)
```
在这个脚本中,我们使用 `import` 语句导入了 `my_module` 模块,并调用了它的 `multiply_numbers` 函数。我们将它们的结果存储在 `result` 变量中,并使用 `print` 函数将结果输出到控制台。
最后,我们可以在终端中运行 `test.py` 文件,它将输出 `6`,这是 `multiply_numbers` 函数对 2 和 3 进行乘法运算的结果。
希望这个示例能帮助你理解如何自定义模块并在其他 Python 源文件中调用它。
阅读全文
相关推荐







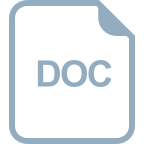






