springboot本地缓存ConcurrentLinkedHashMap实现
时间: 2023-10-16 09:16:58 浏览: 141
Spring Boot 是一个开源的Java框架,它能够帮助开发者快速构建基于Spring的应用程序。为了提高应用程序的性能,Spring Boot提供了本地缓存的支持。本文将介绍如何使用ConcurrentLinkedHashMap实现Spring Boot的本地缓存。
ConcurrentLinkedHashMap是一个线程安全的LRU缓存实现,它基于ConcurrentHashMap和LinkedHashMap。ConcurrentLinkedHashMap提供了以下特性:
1. 线程安全:ConcurrentLinkedHashMap是线程安全的,多个线程可以同时读写缓存。
2. LRU策略:ConcurrentLinkedHashMap使用LRU策略来管理缓存,当缓存满了之后,会将最近最少使用的缓存项删除。
3. 容量控制:ConcurrentLinkedHashMap提供了可配置的容量控制,开发者可以通过设置最大缓存大小来控制缓存的容量。
下面是使用ConcurrentLinkedHashMap实现Spring Boot本地缓存的步骤:
步骤1:添加依赖
在pom.xml文件中添加以下依赖:
```
<dependency>
<groupId>com.googlecode.concurrentlinkedhashmap</groupId>
<artifactId>concurrentlinkedhashmap-lru</artifactId>
<version>1.4.2</version>
</dependency>
```
步骤2:创建缓存类
创建一个缓存类,它包含了ConcurrentLinkedHashMap实例和相关的方法。
```
import com.googlecode.concurrentlinkedhashmap.ConcurrentLinkedHashMap;
import org.springframework.stereotype.Component;
import java.util.Map;
@Component
public class CacheService {
private final Map<String, Object> cache;
public CacheService() {
cache = new ConcurrentLinkedHashMap.Builder<String, Object>()
.maximumWeightedCapacity(1000)
.build();
}
public void put(String key, Object value) {
cache.put(key, value);
}
public Object get(String key) {
return cache.get(key);
}
public void remove(String key) {
cache.remove(key);
}
public void clear() {
cache.clear();
}
}
```
在上面的代码中,我们创建了一个CacheService类,它包含了ConcurrentLinkedHashMap实例和相关的方法。在构造函数中,我们使用ConcurrentLinkedHashMap.Builder创建了一个ConcurrentLinkedHashMap实例,并设置了最大容量为1000。
put、get、remove和clear方法分别用于向缓存中添加、获取、删除和清空数据。
步骤3:使用缓存类
在需要使用缓存的地方,我们可以通过依赖注入的方式获取CacheService实例,并使用其提供的方法进行缓存操作。
```
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class TestController {
@Autowired
private CacheService cacheService;
@GetMapping("/cache/{key}")
public Object cache(@PathVariable String key) {
Object value = cacheService.get(key);
if (value == null) {
// 查询数据库或其他操作
value = "data from db";
cacheService.put(key, value);
}
return value;
}
}
```
在上面的代码中,我们注入了CacheService实例,并在cache方法中使用了它的get和put方法进行缓存操作。如果缓存中不存在指定的key,则查询数据库或其他操作,然后将结果存入缓存中。
这样,我们就使用ConcurrentLinkedHashMap实现了Spring Boot的本地缓存。ConcurrentLinkedHashMap提供了线程安全、LRU策略和容量控制等特性,能够帮助我们提高应用程序的性能。
阅读全文
相关推荐
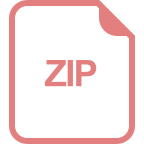
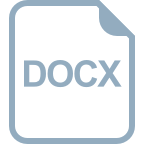
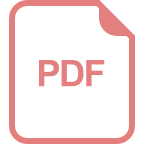
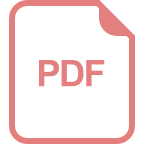
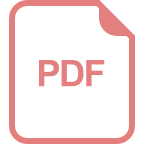
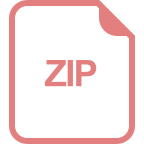
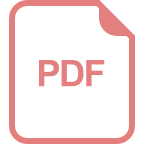
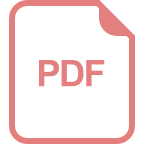
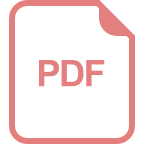
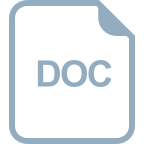
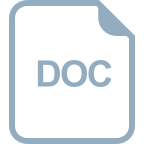
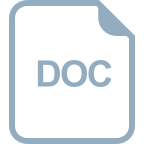
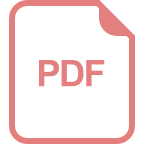
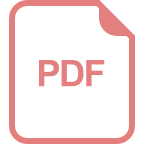
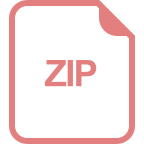