如何在TensorFlow上搭建多层感知机
时间: 2024-02-27 22:59:26 浏览: 142
在TensorFlow上搭建多层感知机(MLP),需要经过以下几个步骤:
1. 导入TensorFlow和其他必要的库:
```python
import tensorflow as tf
import numpy as np
```
2. 定义输入和输出的维度:
```python
input_dim = 784 # 输入维度
hidden_dim = 256 # 隐藏层维度
output_dim = 10 # 输出维度
```
3. 定义输入、输出和隐藏层的占位符:
```python
x = tf.placeholder(tf.float32, [None, input_dim])
y = tf.placeholder(tf.float32, [None, output_dim])
hidden_layer = tf.placeholder(tf.float32, [None, hidden_dim])
```
4. 定义权重和偏置变量:
```python
W1 = tf.Variable(tf.random_normal([input_dim, hidden_dim], stddev=0.1))
b1 = tf.Variable(tf.zeros([hidden_dim]))
W2 = tf.Variable(tf.random_normal([hidden_dim, output_dim], stddev=0.1))
b2 = tf.Variable(tf.zeros([output_dim]))
```
5. 定义前向传播过程:
```python
hidden_layer = tf.nn.relu(tf.matmul(x, W1) + b1)
output_layer = tf.matmul(hidden_layer, W2) + b2
```
6. 定义损失函数和优化器:
```python
loss = tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits(logits=output_layer, labels=y))
optimizer = tf.train.AdamOptimizer().minimize(loss)
```
7. 定义准确率评估:
```python
correct_prediction = tf.equal(tf.argmax(output_layer, 1), tf.argmax(y, 1))
accuracy = tf.reduce_mean(tf.cast(correct_prediction, tf.float32))
```
8. 训练模型并进行预测:
```python
with tf.Session() as sess:
sess.run(tf.global_variables_initializer())
for i in range(num_epochs):
batch_x, batch_y = mnist.train.next_batch(batch_size)
sess.run(optimizer, feed_dict={x: batch_x, y: batch_y})
if i % display_step == 0:
acc = sess.run(accuracy, feed_dict={x: mnist.validation.images, y: mnist.validation.labels})
print("Epoch:", i, "Validation Accuracy:", acc)
test_acc = sess.run(accuracy, feed_dict={x: mnist.test.images, y: mnist.test.labels})
print("Test Accuracy:", test_acc)
```
其中,mnist是一个手写数字识别数据集,可以使用TensorFlow自带的数据集加载器导入。上述代码中,我们使用Adam优化器进行训练,并在每个epoch结束时打印模型在验证集上的准确率,并最终在测试集上评估模型的准确率。
阅读全文
相关推荐
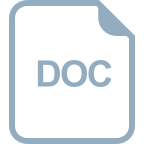
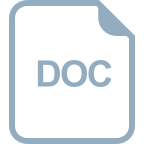
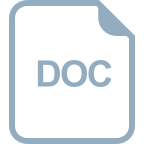
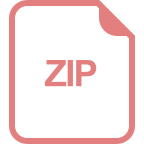

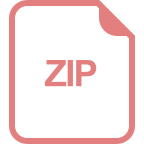
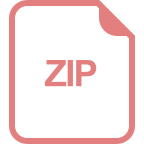
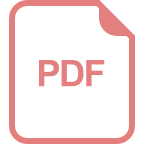
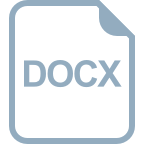
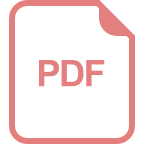
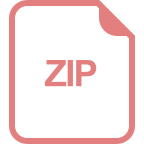
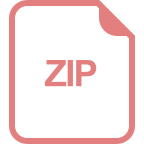
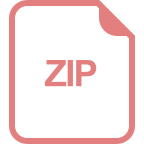
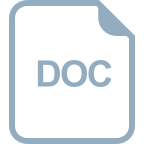
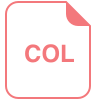
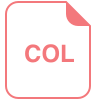


