C#上传 epub的小说 按章节分割 保存数据库 代码实现
时间: 2023-07-27 14:20:28 浏览: 59
好的,以下是一个简单的C#代码示例,演示如何上传epub文件并将其分成章节保存到数据库中:
```csharp
using System;
using System.Data.SqlClient;
using System.IO;
using System.Text.RegularExpressions;
using System.Web;
public partial class UploadEpub : System.Web.UI.Page
{
protected void btnUpload_Click(object sender, EventArgs e)
{
if (fileEpub.HasFile)
{
string fileName = Path.GetFileName(fileEpub.FileName);
string filePath = Server.MapPath("~/EpubFiles/" + fileName);
fileEpub.SaveAs(filePath);
string epubText = GetEpubContent(filePath);
string[] chapters = GetEpubChapters(epubText);
string connectionString = "Data Source=yourServerName;Initial Catalog=yourDatabaseName;Integrated Security=True";
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
SqlCommand command = new SqlCommand();
command.Connection = connection;
// Insert each chapter into database
for (int i = 0; i < chapters.Length; i++)
{
string chapterTitle = GetChapterTitle(chapters[i]);
string chapterContent = GetChapterContent(chapters[i]);
command.CommandText = "INSERT INTO BookChapters (BookTitle, ChapterTitle, ChapterContent) VALUES (@BookTitle, @ChapterTitle, @ChapterContent)";
command.Parameters.AddWithValue("@BookTitle", txtBookTitle.Text);
command.Parameters.AddWithValue("@ChapterTitle", chapterTitle);
command.Parameters.AddWithValue("@ChapterContent", chapterContent);
command.ExecuteNonQuery();
command.Parameters.Clear();
}
connection.Close();
}
}
}
private string GetEpubContent(string filePath)
{
string epubText = "";
using (ZipFile zip = ZipFile.Read(filePath))
{
foreach (ZipEntry entry in zip)
{
if (entry.FileName.EndsWith(".html", StringComparison.OrdinalIgnoreCase))
{
using (MemoryStream stream = new MemoryStream())
{
entry.Extract(stream);
epubText += HttpUtility.HtmlDecode(System.Text.Encoding.UTF8.GetString(stream.ToArray()));
}
}
}
}
return epubText;
}
private string[] GetEpubChapters(string epubText)
{
string pattern = @"<h1>(.*?)</h1>(.*?)<h1>|<h1>(.*?)</h1>(.*?)$";
MatchCollection matches = Regex.Matches(epubText, pattern, RegexOptions.Singleline);
string[] chapters = new string[matches.Count];
for (int i = 0; i < matches.Count; i++)
{
chapters[i] = matches[i].Value;
}
return chapters;
}
private string GetChapterTitle(string chapterText)
{
string pattern = @"<h1>(.*?)</h1>";
Match match = Regex.Match(chapterText, pattern, RegexOptions.Singleline);
return match.Groups[1].Value.Trim();
}
private string GetChapterContent(string chapterText)
{
string pattern = @"<h1>(.*?)</h1>(.*?)<h1>|<h1>(.*?)</h1>(.*?)$";
Match match = Regex.Match(chapterText, pattern, RegexOptions.Singleline);
return match.Groups[2].Value.Trim();
}
}
```
这个例子假设您已经创建了一个名为“BookChapters”的表,用于存储小说的章节。请注意,此代码示例仅供参考,您需要根据自己的需求进行修改和调整。
相关推荐
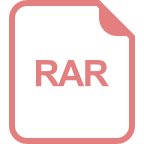














