mybatis分页按照汉字首字母拼音排序
时间: 2023-07-25 18:50:36 浏览: 264
要实现mybatis分页按照汉字首字母拼音排序,可以使用MySQL的内置函数CONVERT将汉字转化为拼音,然后再进行排序。具体实现步骤如下:
1. 在Mapper.xml文件中编写SQL语句,使用CONVERT函数将中文转化为拼音,并进行排序和分页。
```xml
<select id="getUsers" parameterType="map" resultType="User">
SELECT * FROM user ORDER BY CONVERT(name USING gbk) COLLATE gbk_chinese_ci LIMIT #{startIndex}, #{pageSize}
</select>
```
2. 在Mybatis配置文件中添加拦截器,用于在SQL语句执行前设置字符集为gbk。
```xml
<configuration>
<typeAliases>
...
</typeAliases>
<plugins>
<plugin interceptor="com.github.pagehelper.PageInterceptor">
<property name="helperDialect" value="mysql"/>
<property name="reasonable" value="true"/>
</plugin>
<plugin interceptor="com.example.MybatisInterceptor">
<property name="charset" value="gbk"/>
</plugin>
</plugins>
</configuration>
```
3. 编写自定义的MybatisInterceptor类,实现StatementHandler接口,重写prepare方法,在SQL语句执行前设置字符集。
```java
public class MybatisInterceptor implements Interceptor {
private String charset;
public Object intercept(Invocation invocation) throws Throwable {
StatementHandler statementHandler = (StatementHandler) invocation.getTarget();
MetaObject metaObject = SystemMetaObject.forObject(statementHandler);
MappedStatement mappedStatement = (MappedStatement) metaObject.getValue("delegate.mappedStatement");
if (SqlCommandType.SELECT.equals(mappedStatement.getSqlCommandType())) {
BoundSql boundSql = statementHandler.getBoundSql();
String sql = boundSql.getSql();
sql = new String(sql.getBytes("utf-8"), charset);
metaObject.setValue("delegate.boundSql.sql", sql);
}
return invocation.proceed();
}
public Object plugin(Object target) {
return Plugin.wrap(target, this);
}
public void setProperties(Properties properties) {
charset = properties.getProperty("charset", "utf-8");
}
}
```
通过以上步骤,就可以实现mybatis分页按照汉字首字母拼音排序的功能了。
阅读全文
相关推荐
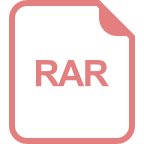
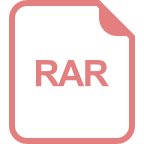
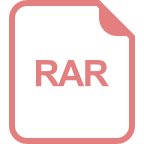
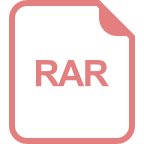
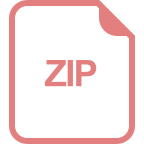
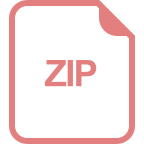
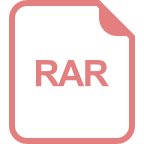
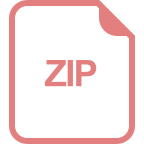
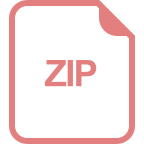
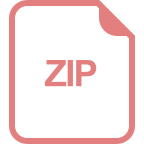
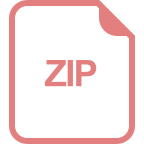
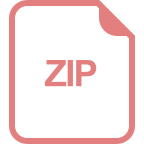
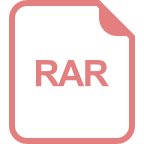