用springboot写一个登录和退出功能,要求有html,js,css,以及功能实现接口,登录要求用html界面输入的用户和密码校验查找数据库数据,再进行登录,并且有拦截器,退出功能 要求退出后清除状态,不能回退
时间: 2023-06-02 13:01:42 浏览: 96
这里提供一个简单的示例,使用Spring Boot、Thymeleaf和Spring Security实现登录和退出功能。
首先,需要创建一个Spring Boot项目,并添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
```
接着,创建一个登录页面`login.html`,代码如下:
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Login</title>
<link rel="stylesheet" th:href="@{/css/login.css}" />
</head>
<body>
<div class="container">
<h1>Login</h1>
<form th:action="@{/login}" method="post">
<input type="text" name="username" placeholder="Username" required="required" />
<input type="password" name="password" placeholder="Password" required="required" />
<button type="submit">Login</button>
</form>
</div>
</body>
</html>
```
在`src/main/resources/static`目录下创建一个`css/login.css`文件,用于美化登录页面的样式,代码如下:
```css
body {
background-color: #f2f2f2;
font-family: 'Open Sans', sans-serif;
}
.container {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
width: 400px;
padding: 40px;
background-color: #fff;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.3);
border-radius: 10px;
}
h1 {
text-align: center;
color: #333;
margin-bottom: 30px;
}
input[type="text"], input[type="password"] {
width: 100%;
padding: 10px;
margin-bottom: 20px;
border-radius: 5px;
border: none;
background-color: #f2f2f2;
color: #333;
font-size: 16px;
}
button[type="submit"] {
display: block;
width: 100%;
padding: 10px;
border-radius: 5px;
border: none;
background-color: #333;
color: #fff;
font-size: 16px;
cursor: pointer;
}
button[type="submit"]:hover {
background-color: #555;
}
```
接下来,创建一个`UserController`类,用于处理登录和退出功能的请求。
```java
@Controller
public class UserController {
@GetMapping("/login")
public String loginPage() {
return "login";
}
@PostMapping("/login")
public String login() {
return "redirect:/home";
}
@GetMapping("/logout")
public String logout(HttpServletRequest request, HttpServletResponse response) {
Authentication auth = SecurityContextHolder.getContext().getAuthentication();
if (auth != null) {
new SecurityContextLogoutHandler().logout(request, response, auth);
}
return "redirect:/login?logout";
}
}
```
在`loginPage`方法中,返回`login`字符串,Spring Boot会自动找到名为`login.html`的页面并返回给客户端。
在`login`方法中,处理登录请求,校验用户名和密码,如果校验通过则重定向到`/home`页面。
在`logout`方法中,处理退出请求,清除当前用户的认证状态,并重定向到`/login?logout`页面。
最后,为了防止用户在退出后通过浏览器回退按钮重新进入系统,需要在`WebSecurityConfig`配置类中添加如下代码:
```java
@Configuration
@EnableWebSecurity
public class WebSecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/login/**").permitAll()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.defaultSuccessUrl("/home")
.permitAll()
.and()
.logout()
.logoutSuccessUrl("/login?logout")
.permitAll()
.and()
.headers().frameOptions().disable()
.and()
.csrf().disable()
.addFilterBefore(new CacheControlFilter(), SecurityContextPersistenceFilter.class)
.addFilterBefore(new CacheControlFilter(), CsrfFilter.class)
.addFilterAfter(new NoCacheFilter(), SecurityContextPersistenceFilter.class)
.addFilterAfter(new NoCacheFilter(), CsrfFilter.class)
.headers().cacheControl().disable();
}
@Autowired
public void configureGlobal(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("admin").password("{noop}admin").roles("ADMIN");
}
}
```
在`configure`方法中,通过`http.headers().cacheControl().disable()`禁用缓存,同时添加了两个过滤器:
- `CacheControlFilter`:设置响应头的缓存策略,禁止客户端缓存。
- `NoCacheFilter`:设置请求头的缓存策略,禁止浏览器缓存。
在`configureGlobal`方法中,添加了一个用户名为`admin`、密码为`admin`、角色为`ADMIN`的用户,用于测试。实际应用中,可以从数据库中动态加载用户信息。
现在,启动应用,访问`http://localhost:8080/login`,即可看到登录页面。输入正确的用户名和密码,点击登录按钮,即可进入`/home`页面。在`/home`页面点击退出按钮,即可退出系统,不能通过浏览器回退按钮重新进入系统。
相关推荐
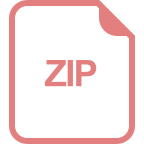
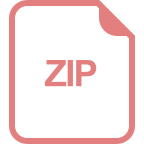
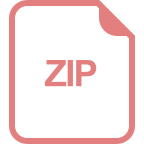
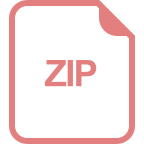
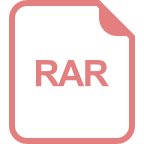
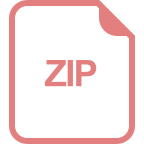
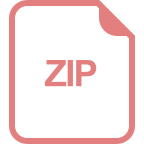
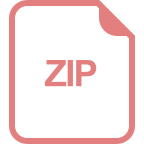
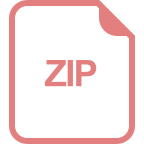
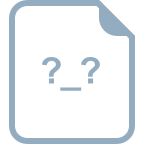
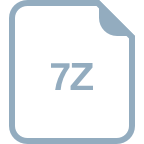