js 当前时间减两天
时间: 2023-08-31 17:39:36 浏览: 37
### 回答1:
可以使用 JavaScript 中的 Date 对象来实现。以下是将当前时间减去两天的代码示例:
```javascript
var today = new Date();
today.setDate(today.getDate() - 2);
console.log(today);
```
这个代码片段中,首先创建了一个 Date 对象来表示当前时间,然后使用 setDate() 方法将日期设置为当前日期减去两天。最后,通过 console.log() 打印出新的日期对象。
### 回答2:
在JavaScript中,我们可以使用`Date`对象和`getTime()`方法来实现将当前时间减去两天的操作。下面是具体步骤:
1. 首先,我们创建一个`Date`对象来获取当前的日期和时间:
```javascript
var currentDate = new Date();
```
2. 通过`getTime()`方法,我们可以获得当前日期的时间戳:
```javascript
var currentTime = currentDate.getTime();
```
3. 获取两天的毫秒数:
```javascript
var twoDays = 2 * 24 * 60 * 60 * 1000;
```
4. 将当前时间减去两天的毫秒数:
```javascript
var newTime = currentTime - twoDays;
```
5. 最后,我们可以用新的时间戳创建一个新的`Date`对象来表示减去两天的日期:
```javascript
var newDate = new Date(newTime);
```
现在,`newDate`就是当前时间减去两天后的日期。
完整的代码如下所示:
```javascript
var currentDate = new Date();
var currentTime = currentDate.getTime();
var twoDays = 2 * 24 * 60 * 60 * 1000;
var newTime = currentTime - twoDays;
var newDate = new Date(newTime);
```
希望这个答案能够帮助到你!
### 回答3:
要将当前时间减去两天,我们可以使用JavaScript中的Date对象和相关方法来实现。
首先,我们可以使用Date对象的构造函数来获取当前时间的日期和时间。
```javascript
var currentDate = new Date();
```
接下来,我们可以使用Date对象的setDate()方法来将日期减去两天。
```javascript
currentDate.setDate(currentDate.getDate() - 2);
```
最后,我们可以使用Date对象的各种方法来获取和格式化当前时间的日期和时间。
```javascript
var year = currentDate.getFullYear();
var month = currentDate.getMonth() + 1;
var day = currentDate.getDate();
var hours = currentDate.getHours();
var minutes = currentDate.getMinutes();
var seconds = currentDate.getSeconds();
// 将月份、日期、小时、分钟、秒数小于10的数字前面加上0
month = month < 10 ? '0' + month : month;
day = day < 10 ? '0' + day : day;
hours = hours < 10 ? '0' + hours : hours;
minutes = minutes < 10 ? '0' + minutes : minutes;
seconds = seconds < 10 ? '0' + seconds : seconds;
// 格式化当前时间
var formattedCurrentTime = year + '-' + month + '-' + day + ' ' + hours + ':' + minutes + ':' + seconds;
// 输出结果
console.log("当前时间减去两天后的日期和时间为:" + formattedCurrentTime);
```
这样,我们就可以得到当前时间减去两天后的日期和时间。
相关推荐
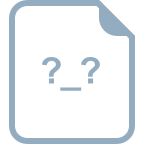
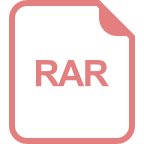














