outputstandard output You are given a string s consisting of the characters 0, 1 and/or ?. Let's call it a pattern. Let's say that the binary string (a string where each character is either 0 or 1) matches the pattern if you can replace each character ? with 0 or 1 (for each character, the choice is independent) so that the strings become equal. For example, 0010 matches ?01?, but 010 doesn't match 1??, ??, or ????. Let's define the cost of the binary string as the minimum number of operations of the form "reverse an arbitrary contiguous substring of the string" required to sort the string in non-descending order. You have to find a binary string with the minimum possible cost among those that match the given pattern. If there are multiple answers, print any of them.
时间: 2024-01-17 20:03:32 浏览: 131
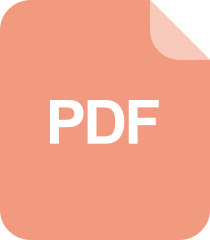
The Implementation of Multilevel Green's Function Interpolation Method for Full-Wave Electromagnetic Problems
This is an interesting problem! To solve it, you can use dynamic programming. Let dp[i][j] be the minimum cost to sort the first i characters of the pattern such that the i-th character is j (0 or 1). The base case is dp[0][0]=dp[0][1]=0, since an empty string is already sorted.
Then, to compute dp[i][j], you can consider the last character of the pattern, which can be either 0, 1, or ?. If it's 0 or 1, you can simply match it with the i-th character of the binary string, and update dp[i][j] accordingly. If it's ?, you need to try both possibilities (0 or 1) and take the minimum cost.
The final answer is the minimum of dp[n][0] and dp[n][1], where n is the length of the pattern.
Here's the Python code:
```
s = input().strip()
n = len(s)
dp = [[float('inf')]*2 for _ in range(n+1)]
dp[0][0] = dp[0][1] = 0
for i in range(1, n+1):
if s[i-1] == '0':
dp[i][0] = min(dp[i-1][0], dp[i-1][1]+1)
elif s[i-1] == '1':
dp[i][1] = min(dp[i-1][1], dp[i-1][0]+1)
else:
dp[i][0] = min(dp[i-1][0], dp[i-1][1]+1)
dp[i][1] = min(dp[i-1][1], dp[i-1][0]+1)
ans = [''] * n
if dp[n][0] <= dp[n][1]:
i, j = n, 0
while i > 0:
if s[i-1] == '0':
ans[i-1] = '0'
i, j = i-1, 0
elif s[i-1] == '1':
ans[i-1] = '1'
i, j = i-1, 1
else:
if dp[i][j] == dp[i-1][0]:
ans[i-1] = '0'
i, j = i-1, 0
else:
ans[i-1] = '1'
i, j = i-1, 1
else:
i, j = n, 1
while i > 0:
if s[i-1] == '0':
ans[i-1] = '0'
i, j = i-1, 0
elif s[i-1] == '1':
ans[i-1] = '1'
i, j = i-1, 1
else:
if dp[i][j] == dp[i-1][1]:
ans[i-1] = '1'
i, j = i-1, 1
else:
ans[i-1] = '0'
i, j = i-1, 0
print(''.join(ans))
```
Hope this helps!
阅读全文
相关推荐
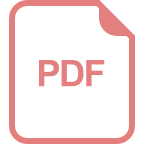
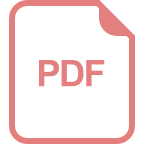














