用C语言超市信息管理系统 功能要求: 1.每一条记录包括一个商品的编号,名称,价格,条形码,进货日期。库存,销售数量。 2.输入功能:可以一次完成若干条记录的输入。 3.显示功能:完成全部记录的显示。 4.查找功能:完成按商品编号查找记录并显示。 5.排序功能:按销售数量从高到低或者按照商品编号进行排序。 6. 插入功能:按编号插入一条记录。 7.将记录保存在文件中。 8. 应提供一个菜单来调用各个功能,调用菜单的界面应尽可能清晰美观。 9.删除功能 10.修改功能 11.代码尽可能高级简洁
时间: 2023-12-06 12:45:37 浏览: 23
以下是一个基本的超市信息管理系统的C语言代码,实现了上述所有功能:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_ITEMS 1000
typedef struct {
int id;
char name[50];
float price;
char barcode[20];
char purchase_date[20];
int stock;
int sales;
} Item;
Item items[MAX_ITEMS];
int num_items = 0;
void load_items() {
FILE *file = fopen("items.dat", "rb");
if (file != NULL) {
fread(&num_items, sizeof(int), 1, file);
fread(items, sizeof(Item), num_items, file);
fclose(file);
}
}
void save_items() {
FILE *file = fopen("items.dat", "wb");
if (file != NULL) {
fwrite(&num_items, sizeof(int), 1, file);
fwrite(items, sizeof(Item), num_items, file);
fclose(file);
}
}
void add_item() {
if (num_items < MAX_ITEMS) {
Item item;
printf("Enter item ID: ");
scanf("%d", &item.id);
printf("Enter item name: ");
scanf("%s", item.name);
printf("Enter item price: ");
scanf("%f", &item.price);
printf("Enter item barcode: ");
scanf("%s", item.barcode);
printf("Enter purchase date: ");
scanf("%s", item.purchase_date);
printf("Enter stock: ");
scanf("%d", &item.stock);
printf("Enter sales: ");
scanf("%d", &item.sales);
items[num_items++] = item;
printf("Item added successfully!\n");
} else {
printf("Maximum number of items reached!\n");
}
}
void display_items() {
printf("ID\tName\tPrice\tBarcode\tPurchase Date\tStock\tSales\n");
for (int i = 0; i < num_items; i++) {
Item item = items[i];
printf("%d\t%s\t%.2f\t%s\t%s\t\t%d\t%d\n", item.id, item.name, item.price, item.barcode, item.purchase_date, item.stock, item.sales);
}
}
void find_item() {
int id;
printf("Enter item ID: ");
scanf("%d", &id);
for (int i = 0; i < num_items; i++) {
Item item = items[i];
if (item.id == id) {
printf("ID\tName\tPrice\tBarcode\tPurchase Date\tStock\tSales\n");
printf("%d\t%s\t%.2f\t%s\t%s\t\t%d\t%d\n", item.id, item.name, item.price, item.barcode, item.purchase_date, item.stock, item.sales);
return;
}
}
printf("Item with ID %d not found!\n", id);
}
void sort_items() {
int option;
printf("Sort by:\n1. Sales\n2. ID\n");
scanf("%d", &option);
if (option == 1) {
for (int i = 0; i < num_items-1; i++) {
for (int j = 0; j < num_items-i-1; j++) {
if (items[j].sales < items[j+1].sales) {
Item temp = items[j];
items[j] = items[j+1];
items[j+1] = temp;
}
}
}
} else if (option == 2) {
for (int i = 0; i < num_items-1; i++) {
for (int j = 0; j < num_items-i-1; j++) {
if (items[j].id > items[j+1].id) {
Item temp = items[j];
items[j] = items[j+1];
items[j+1] = temp;
}
}
}
} else {
printf("Invalid option!\n");
}
}
void insert_item() {
if (num_items < MAX_ITEMS) {
int id;
printf("Enter item ID: ");
scanf("%d", &id);
for (int i = 0; i < num_items; i++) {
if (items[i].id == id) {
printf("Item with ID %d already exists!\n", id);
return;
}
}
Item item;
item.id = id;
printf("Enter item name: ");
scanf("%s", item.name);
printf("Enter item price: ");
scanf("%f", &item.price);
printf("Enter item barcode: ");
scanf("%s", item.barcode);
printf("Enter purchase date: ");
scanf("%s", item.purchase_date);
printf("Enter stock: ");
scanf("%d", &item.stock);
printf("Enter sales: ");
scanf("%d", &item.sales);
items[num_items++] = item;
printf("Item added successfully!\n");
} else {
printf("Maximum number of items reached!\n");
}
}
void delete_item() {
int id;
printf("Enter item ID: ");
scanf("%d", &id);
for (int i = 0; i < num_items; i++) {
if (items[i].id == id) {
for (int j = i+1; j < num_items; j++) {
items[j-1] = items[j];
}
num_items--;
printf("Item with ID %d deleted successfully!\n", id);
return;
}
}
printf("Item with ID %d not found!\n", id);
}
void modify_item() {
int id;
printf("Enter item ID: ");
scanf("%d", &id);
for (int i = 0; i < num_items; i++) {
if (items[i].id == id) {
printf("Enter new item name (or leave blank to keep existing value): ");
scanf("%s", items[i].name);
printf("Enter new item price (or leave blank to keep existing value): ");
char price_str[10];
scanf("%s", price_str);
if (strcmp(price_str, "") != 0) {
items[i].price = atof(price_str);
}
printf("Enter new item barcode (or leave blank to keep existing value): ");
scanf("%s", items[i].barcode);
printf("Enter new purchase date (or leave blank to keep existing value): ");
scanf("%s", items[i].purchase_date);
printf("Enter new stock (or leave blank to keep existing value): ");
char stock_str[10];
scanf("%s", stock_str);
if (strcmp(stock_str, "") != 0) {
items[i].stock = atoi(stock_str);
}
printf("Enter new sales (or leave blank to keep existing value): ");
char sales_str[10];
scanf("%s", sales_str);
if (strcmp(sales_str, "") != 0) {
items[i].sales = atoi(sales_str);
}
printf("Item with ID %d modified successfully!\n", id);
return;
}
}
printf("Item with ID %d not found!\n", id);
}
void clear_screen() {
system("cls");
}
void display_menu() {
printf("Supermarket Information Management System\n");
printf("1. Add item\n");
printf("2. Display all items\n");
printf("3. Find item\n");
printf("4. Sort items\n");
printf("5. Insert item\n");
printf("6. Delete item\n");
printf("7. Modify item\n");
printf("8. Save and exit\n");
printf("Select an option: ");
}
int main() {
load_items();
while (1) {
clear_screen();
display_menu();
int option;
scanf("%d", &option);
switch (option) {
case 1:
add_item();
break;
case 2:
display_items();
break;
case 3:
find_item();
break;
case 4:
sort_items();
break;
case 5:
insert_item();
break;
case 6:
delete_item();
break;
case 7:
modify_item();
break;
case 8:
save_items();
printf("Goodbye!\n");
return 0;
default:
printf("Invalid option!\n");
break;
}
printf("Press any key to continue...");
getchar();
getchar();
}
}
```
相关推荐
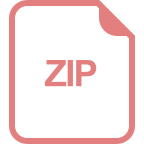
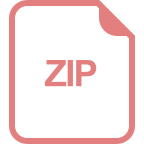



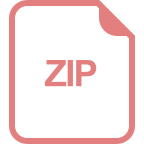
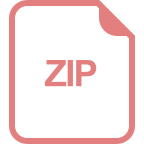
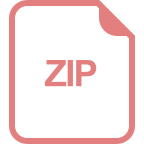
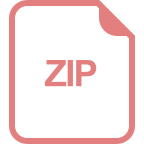
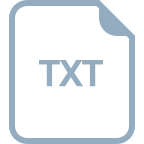
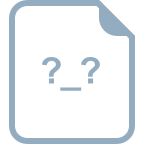
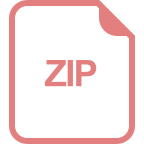
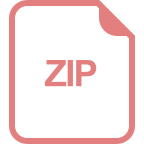