c语言: ifstream.open()什么意思和要怎么用
时间: 2023-06-04 10:04:42 浏览: 354
ifstream.open() 是 c++ 中用于打开文件的函数。它可以接受一个文件名或路径作为参数来打开文件,并提供不同的打开文件模式,例如只读、只写、二进制、文本等。使用 ifstream.open() 函数打开文件后,你就可以使用其他函数像读取文件、写入数据等操作。下面是一个示例代码:
```
#include <iostream>
#include <fstream>
using namespace std;
int main() {
ifstream file("example.txt");
if (file.is_open()) {
string line;
while (getline(file,line)) {
cout << line << '\n';
}
file.close();
} else {
cout << "Unable to open file\n";
}
return 0;
}
```
这个例子会打开名为“example.txt”的文件并逐行读取它,最后关闭文件。如果文件无法打开,则会输出“Unable to open file”错误信息。
相关问题
C语言 ifstream和ofstream的区别是什么,可以举例一下相关的内容
C++中的ifstream和ofstream用于文件的输入输出操作。它们是iostream类的子类,分别表示输入流和输出流。它们的主要区别在于:
1. ifstream是从文件中读取数据,而ofstream是向文件中写入数据。
2. ifstream打开文件时使用“ifstream”类,而ofstream打开文件时使用“ofstream”类。
3. ifstream使用“>>”操作符从文件中读取数据,而ofstream使用“<<”操作符向文件中写入数据。
下面是一个使用ifstream和ofstream的简单示例:
```
#include <iostream>
#include <fstream>
using namespace std;
int main() {
// 从文件中读取数据
ifstream input_file;
input_file.open("input.txt");
int x;
input_file >> x;
input_file.close();
cout << "读取到的数字为:" << x << endl;
// 向文件中写入数据
ofstream output_file;
output_file.open("output.txt");
output_file << "Hello, World!" << endl;
output_file.close();
return 0;
}
```
在该示例中,我们使用ifstream读取一个整数并将其存储在变量x中,然后使用ofstream将一条消息写入名为“output.txt”的文件中。
c语言ifstream
C语言中没有ifstream这个关键字。ifstream是C++中的输入文件流类,用于读取文件内容。如果你想在C语言中读取文件内容,可以使用标准库函数fopen和fread来实现。以下是一个使用fopen和fread读取文件内容的示例:
```c
#include <stdio.h>
int main() {
FILE *file = fopen("filename.txt", "rb");
if (file == NULL) {
printf("Failed to open file.\n");
return 1;
}
fseek(file, 0, SEEK_END);
long file_size = ftell(file);
fseek(file, 0, SEEK_SET);
char *buffer = malloc(file_size + 1);
if (buffer == NULL) {
printf("Failed to allocate memory.\n");
fclose(file);
return 1;
}
fread(buffer, file_size, 1, file);
buffer[file_size] = '\0';
printf("File content: %s\n", buffer);
free(buffer);
fclose(file);
return 0;
}
```
在上面的代码中,我们首先使用fopen函数打开文件,并指定打开模式为"rb",表示以二进制方式读取文件。然后使用fseek和ftell函数来获取文件大小,为了读取整个文件内容,我们将文件指针移动到文件末尾,然后使用ftell函数获取文件大小,再将文件指针移回到文件开头。接下来,我们使用malloc函数动态分配内存来存储文件内容,然后使用fread函数读取文件内容到内存中。最后,我们打印出文件内容,并释放内存和关闭文件。
请注意,以上示例是使用C语言标准库函数来读取文件内容的一种方式,如果你需要更多的文件操作功能,可以查阅C语言的文件操作相关文档。
相关推荐
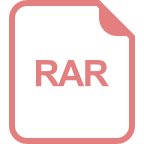
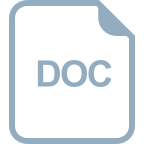












