定义一个“点”类Point,并在此基础上定义长方形类Rect以及圆类Circle,并求出派生类的周长和面积(自行设计成员变量和成员函数)。
时间: 2023-07-10 22:20:58 浏览: 52
好的,下面是代码实现:
```c++
class Point {
protected:
double x, y;
public:
Point(double x = 0, double y = 0) : x(x), y(y) {}
};
class Rect : public Point {
private:
double width, height;
public:
Rect(double x = 0, double y = 0, double width = 0, double height = 0) : Point(x, y), width(width), height(height) {}
double getPerimeter() {
return 2 * (width + height);
}
double getArea() {
return width * height;
}
};
class Circle : public Point {
private:
double radius;
public:
Circle(double x = 0, double y = 0, double radius = 0) : Point(x, y), radius(radius) {}
double getPerimeter() {
return 2 * 3.14 * radius;
}
double getArea() {
return 3.14 * radius * radius;
}
};
```
以上代码定义了一个基类Point,表示平面上的一个点。然后定义了两个派生类Rect和Circle,分别表示长方形和圆形。在Rect和Circle中,通过继承Point类来获得x和y坐标,并添加了自己的成员变量width、height、radius来表示长方形和圆形的特征。同时还定义了getPerimeter()和getArea()成员函数,分别用于计算周长和面积。
使用示例:
```c++
int main() {
Rect r(1, 1, 2, 3);
Circle c(1, 1, 2);
cout << "Rect perimeter:" << r.getPerimeter() << endl;
cout << "Rect area:" << r.getArea() << endl;
cout << "Circle perimeter:" << c.getPerimeter() << endl;
cout << "Circle area:" << c.getArea() << endl;
return 0;
}
```
输出结果:
```
Rect perimeter:10
Rect area:6
Circle perimeter:12.56
Circle area:12.56
```
可以看到,Rect和Circle都成功的计算出了自己的周长和面积。
相关推荐
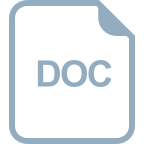














