使用numpy读取xlsx文件并生成.h头文件
时间: 2023-09-21 08:05:46 浏览: 216
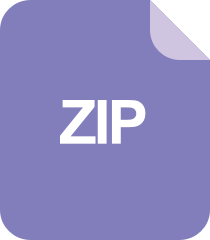
numpy的HTML格式文档
### 回答1:
使用 numpy 读取 xlsx 文件并生成 .h 头文件的步骤如下:
1. 首先,你需要安装 numpy 和 pandas 库。你可以使用 pip 命令来安装:
```
pip install numpy
pip install pandas
```
2. 然后,使用 pandas 库的 read_excel() 函数读取 xlsx 文件。例如:
```
import pandas as pd
df = pd.read_excel('myfile.xlsx')
```
3. 接下来,使用 numpy 库的 savetxt() 函数将读取到的数据保存到 .h 头文件中。例如:
```
import numpy as np
np.savetxt('myfile.h', df.values, fmt='%d', delimiter=',')
```
这样就可以将 xlsx 文件中的数据读取到 numpy 数组中,并将其保存到 .h 头文件中了。
注意:在这个例子中,我们假设你的 xlsx 文件中的数据都是整数,因此我们使用了 fmt='%d' 参数。如果你的数据包含浮点数或其他类型的数据,你需要使用对应的格式字符串。
### 回答2:
为了使用numpy读取xlsx文件并生成.h头文件,首先需要安装numpy库和pandas库。
安装numpy和pandas库的方法是使用命令行执行以下命令:
```
pip install numpy
pip install pandas
```
安装完毕后,我们可以使用以下代码来读取xlsx文件并生成.h头文件:
```python
import pandas as pd
import numpy as np
# 读取xlsx文件
data = pd.read_excel('filename.xlsx')
# 将读取到的数据转换为numpy数组
array_data = np.array(data)
# 生成.h头文件
output_file = open('output.h', 'w')
output_file.write("#ifndef HEADER_H\n")
output_file.write("#define HEADER_H\n")
output_file.write("\n")
output_file.write("const int rows = %d;\n" % array_data.shape[0])
output_file.write("const int cols = %d;\n" % array_data.shape[1])
output_file.write("\n")
output_file.write("int data[rows][cols] = {\n")
# 将数组数据写入.h文件中
for i in range(array_data.shape[0]):
output_file.write(" {")
for j in range(array_data.shape[1]):
output_file.write("%d" % array_data[i][j])
if j != array_data.shape[1] - 1:
output_file.write(", ")
output_file.write("}")
if i != array_data.shape[0] - 1:
output_file.write(",")
output_file.write("\n")
output_file.write("};\n\n")
output_file.write("#endif\n")
output_file.close()
```
在这个代码中,我们先使用pandas的`read_excel`函数读取xlsx文件,并将结果保存在一个DataFrame对象中。然后,我们使用numpy的`array`函数将DataFrame对象转换为numpy数组。最后,我们将数组数据写入一个.h头文件中,该头文件中包含了一些必要的定义,如数据的行数、列数等。
将以上代码保存为一个python文件,运行该文件即可生成对应的.h头文件。需要确保在同一目录下有一个名为"filename.xlsx"的xlsx文件。生成的.h头文件名为"output.h"。
上述代码可以根据实际情况进行修改。如果xlsx文件的内容不是整数,可以根据需要进行相应修改。
### 回答3:
使用NumPy读取xlsx文件并生成.h头文件的步骤如下:
首先,安装所需的Python库:NumPy和pandas。可以使用以下命令在终端或命令提示符中安装它们:
```
pip install numpy
pip install pandas
```
然后,在Python脚本中导入所需的库:
```python
import numpy as np
import pandas as pd
```
接下来,使用pandas的`read_excel`函数读取xlsx文件并将其转换为NumPy数组:
```python
df = pd.read_excel('example.xlsx') # 读取xlsx文件
data = df.to_numpy() # 将数据转换为NumPy数组
```
然后,将转换后的NumPy数组保存为.h头文件,以便在其他程序中使用:
```python
header_file = open('data.h', 'w') # 打开.h头文件
header_file.write('#ifndef DATA_H\n') # 写入头文件保护宏
header_file.write('#define DATA_H\n\n')
# 根据NumPy数组的形状和元素逐行写入.h头文件
header_file.write('const int data[{}][{}] = {{\n'.format(data.shape[0], data.shape[1]))
for row in data:
header_file.write('\t{')
for i in range(len(row)-1):
header_file.write(str(row[i]) + ', ')
header_file.write(str(row[-1]) + '},\n')
header_file.write('};\n\n')
header_file.write('#endif') # 写入头文件结尾的#endif
header_file.close() # 关闭文件
```
最后,生成的.h头文件中将包含NumPy数组的数据。可以在其他的C或C++项目中使用这个头文件。
注意:上述代码中的`example.xlsx`应替换为实际的xlsx文件路径和文件名。如果需要将其他信息从xlsx文件中读取到.h头文件中,可以修改代码以满足需求。
阅读全文
相关推荐
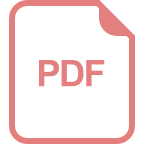

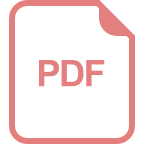
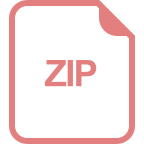
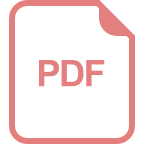
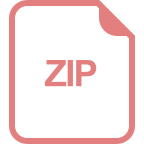
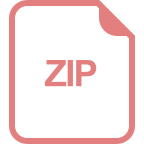
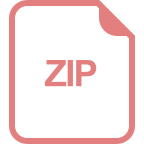
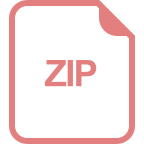
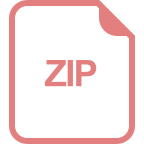
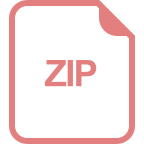
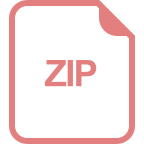
