帮我用java语言洗一个自动生成迷宫的代码
时间: 2023-06-08 18:08:04 浏览: 52
好的,有关用java语言洗一个自动生成迷宫的代码,可以参考以下代码:
```java
import java.util.*;
public class MazeGenerator {
private final int[][] maze;
private final int width;
private final int height;
private final Random random = new Random();
public MazeGenerator(int width, int height) {
this.width = width;
this.height = height;
maze = new int[width][height];
generateMaze(0, 0);
}
public int[][] getMaze() {
return maze;
}
private void generateMaze(int x, int y) {
List<Direction> directions = Arrays.asList(Direction.values());
Collections.shuffle(directions, random);
for (Direction direction : directions) {
int newX = x + direction.dx;
int newY = y + direction.dy;
if (between(newX, width) && between(newY, height) && (maze[newX][newY] == 0)) {
maze[x + direction.dx / 2][y + direction.dy / 2] = 1;
generateMaze(newX, newY);
}
}
}
private boolean between(int v, int upper) {
return (v >= 0) && (v < upper);
}
private enum Direction {
NORTH(0, -1),
EAST(1, 0),
SOUTH(0, 1),
WEST(-1, 0);
int dx;
int dy;
Direction(int dx, int dy) {
this.dx = dx;
this.dy = dy;
}
}
public static void main(String[] args) {
int width = 10;
int height = 10;
MazeGenerator mazeGenerator = new MazeGenerator(width, height);
int[][] maze = mazeGenerator.getMaze();
for (int x = 0; x < width; x++) {
// draw the north edge
for (int y = 0; y < height; y++) {
System.out.print((maze[x][y] & 1) == 0 ? "+ " : "+---");
}
System.out.println("+");
// draw the west edge
for (int y = 0; y < height; y++) {
System.out.print((maze[x][y] & 8) == 0 ? "| " : " ");
}
System.out.println("|");
}
// draw the bottom line
for (int y = 0; y < height; y++) {
System.out.print("+---");
}
System.out.println("+");
}
}
```
这段代码可以生成一个宽为 width,高为 height 的迷宫。使用数组 maze 表示迷宫,0 表示墙壁,1 表示通路。generateMaze 方法使用递归方法生成迷宫,between 方法用于判断当前位置是否超出迷宫的边界。输出使用 ASCII 字符画绘制迷宫。希望这段代码对您有所帮助!
相关推荐
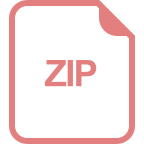
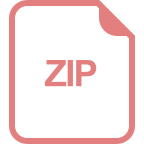
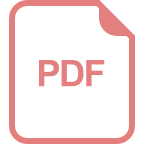














