编写程序,用系统调用fork()创建两子进程。父进程显示10次字符串“father”,子进程1显示10次字符串“son”,子进程2显示10次字符串“daughter”。
时间: 2024-05-12 13:17:49 浏览: 128
好的,这是一个很简单的任务,您可以使用以下代码来实现:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/wait.h>
void child_process(char *str) {
for (int i = 0; i < 10; i++) {
printf("%s\n", str);
sleep(1);
}
exit(0);
}
int main() {
pid_t pid1, pid2;
// Create 1st child process
pid1 = fork();
if (pid1 == 0) {
child_process("son");
} else if (pid1 < 0) {
perror("fork");
exit(1);
}
// Create 2nd child process
pid2 = fork();
if (pid2 == 0) {
child_process("daughter");
} else if (pid2 < 0) {
perror("fork");
exit(1);
}
// Father process
for (int i = 0; i < 10; i++) {
printf("father\n");
sleep(1);
}
// Wait for both child processes to finish
waitpid(pid1, NULL, 0);
waitpid(pid2, NULL, 0);
return 0;
}
```
请注意,此代码并未进行错误检查和错误处理,为了简化此示例,我们在此忽略了这些步骤。
阅读全文
相关推荐
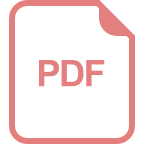
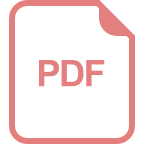
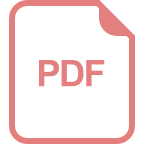





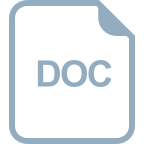
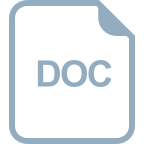
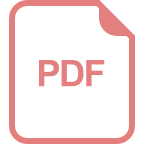
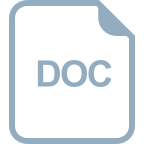
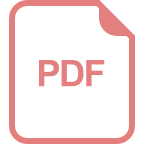
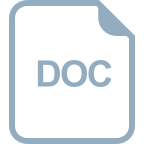
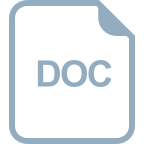
