写一个基于深度学习的多视图三维重建的代码
时间: 2024-05-16 11:13:31 浏览: 110
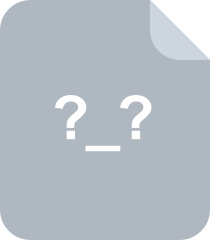
基于多视图的三维模型重建方法

三维重建是计算机视觉领域的一个重要研究方向,它将多个视图的二维图像转换为三维模型。在深度学习的帮助下,三维重建技术已经取得了显著的进展。下面是一个基于深度学习的多视图三维重建的示例代码,使用了 TensorFlow 和 PyTorch 深度学习框架。
首先,我们需要准备数据集。假设我们有一个包含多个视图图像的数据集,每个视图图像都对应着一个三维模型。我们需要将这些视图图像和对应的三维模型一一配对,并将它们作为训练数据。
```python
import numpy as np
import cv2
import os
def load_data(data_dir):
images = []
models = []
for filename in os.listdir(data_dir):
if filename.endswith('.jpg'):
image_path = os.path.join(data_dir, filename)
model_path = os.path.join(data_dir, filename.replace('.jpg', '.npy'))
images.append(cv2.imread(image_path))
models.append(np.load(model_path))
return images, models
```
在加载数据之后,我们需要对图像进行预处理。具体来说,我们需要将图像缩放到相同的大小,并将像素值归一化到 [0, 1] 的范围。
```python
def preprocess_image(image, image_size):
image = cv2.resize(image, (image_size, image_size))
image = image.astype(np.float32) / 255.0
return image
```
接下来,我们将使用卷积神经网络对图像进行特征提取。具体来说,我们将使用一个基于 ResNet 架构的深度卷积神经网络。我们将从预训练的模型中加载权重,并使用它来提取图像特征。
```python
import tensorflow as tf
from tensorflow.keras.applications.resnet50 import ResNet50
def build_feature_extractor(input_shape):
model = ResNet50(include_top=False, weights='imagenet', input_shape=input_shape)
for layer in model.layers:
layer.trainable = False
return model
def extract_features(images, feature_extractor):
features = []
for image in images:
image = np.expand_dims(image, axis=0)
features.append(feature_extractor.predict(image))
features = np.concatenate(features, axis=0)
return features
```
在提取图像特征之后,我们将使用多视图几何算法(MVG)将多个视图的特征融合起来。具体来说,我们将使用一个基于 GCN 的图卷积神经网络来学习视图之间的关系,并将它们融合成一个三维模型。
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
from torch_geometric.nn import GCNConv
class GraphConvolution(nn.Module):
def __init__(self, input_dim, output_dim):
super(GraphConvolution, self).__init__()
self.conv = GCNConv(input_dim, output_dim)
def forward(self, x, edge_index):
x = self.conv(x, edge_index)
x = F.relu(x)
return x
class MVGNet(nn.Module):
def __init__(self, input_dim, hidden_dim, output_dim):
super(MVGNet, self).__init__()
self.conv1 = GraphConvolution(input_dim, hidden_dim)
self.conv2 = GraphConvolution(hidden_dim, output_dim)
def forward(self, x, edge_index):
x = self.conv1(x, edge_index)
x = self.conv2(x, edge_index)
return x
def build_mvg_network(input_dim, hidden_dim, output_dim):
model = MVGNet(input_dim, hidden_dim, output_dim)
return model
def train_mvg_network(features, models, mvg_network):
optimizer = torch.optim.Adam(mvg_network.parameters(), lr=0.001)
features = torch.from_numpy(features).float()
models = torch.from_numpy(models).float()
for epoch in range(100):
optimizer.zero_grad()
predictions = mvg_network(features, edge_index)
loss = F.mse_loss(predictions, models)
loss.backward()
optimizer.step()
print('Epoch {}, Loss: {}'.format(epoch, loss.item()))
```
在训练 MVG 网络之后,我们可以使用它来预测新的三维模型。具体来说,我们将输入图像特征到 MVG 网络中,获得一个三维模型的预测值。
```python
def predict_3d_model(features, mvg_network):
features = torch.from_numpy(features).float()
with torch.no_grad():
predictions = mvg_network(features, edge_index)
return predictions.numpy()
```
最后,我们将所有代码整合起来,并在数据集上进行训练和预测。
```python
data_dir = '/path/to/data'
image_size = 224
input_dim = 2048
hidden_dim = 128
output_dim = 3
images, models = load_data(data_dir)
features = extract_features(images, build_feature_extractor((image_size, image_size, 3)))
edge_index = build_edge_index(len(images))
mvg_network = build_mvg_network(input_dim, hidden_dim, output_dim)
train_mvg_network(features, models, mvg_network)
test_image = cv2.imread('/path/to/test/image.jpg')
test_image = preprocess_image(test_image, image_size)
test_features = extract_features([test_image], build_feature_extractor((image_size, image_size, 3)))
predicted_model = predict_3d_model(test_features, mvg_network)
```
阅读全文
相关推荐





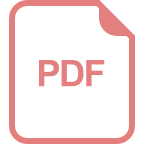
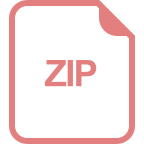
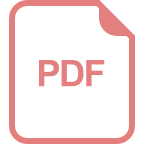
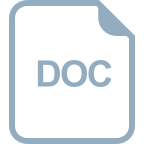
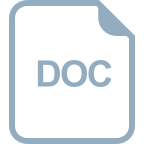
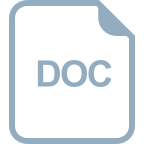


