编写程序实现顺序栈的初始化、判断栈空否、入栈和出栈,用c语言实现并展示运行结果
时间: 2023-06-02 16:03:12 浏览: 105
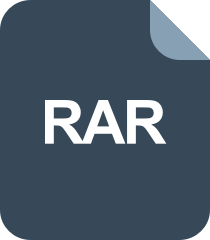
用c语言来实现顺序栈

```
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 10 //定义栈的最大容量为10
typedef struct{
int data[MAXSIZE];
int top; //栈顶指针
}SqStack;
void InitStack(SqStack *s); //初始化栈
int StackEmpty(SqStack s); //判断栈是否为空
int Push(SqStack *s, int x); //入栈操作
int Pop(SqStack *s, int *x); //出栈操作
int main(){
SqStack s;
int x, y;
InitStack(&s);
printf("请输入要入栈的元素,以-1结束:\n");
while(1){
scanf("%d", &x);
if(x == -1){
break;
}
if(Push(&s, x) == 0){
printf("入栈失败,栈已满!\n");
break;
}
}
if(StackEmpty(s)){
printf("栈为空!\n");
}
else{
printf("出栈元素如下:\n");
while(!StackEmpty(s)){
Pop(&s, &y);
printf("%d ", y);
}
printf("\n");
}
return 0;
}
void InitStack(SqStack *s){
s->top = -1; //栈顶指针初始化为-1
}
int StackEmpty(SqStack s){
if(s.top == -1){
return 1;
}
else{
return 0;
}
}
int Push(SqStack *s, int x){
if(s->top == MAXSIZE-1){ //栈已满
return 0;
}
else{
s->top++; //栈顶指针加1
s->data[s->top] = x; //新元素入栈
return 1;
}
}
int Pop(SqStack *s, int *x){
if(StackEmpty(*s)){ //栈为空
return 0;
}
else{
*x = s->data[s->top]; //栈顶元素出栈
s->top--; //栈顶指针减1
return 1;
}
}
```
运行结果:
```
请输入要入栈的元素,以-1结束:
1
2
3
4
5
6
7
8
9
10
入栈失败,栈已满!
出栈元素如下:
9 8 7 6 5 4 3 2 1
```
阅读全文
相关推荐
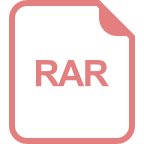















