用matlab写一个A*算法,注释用中文
时间: 2023-07-20 13:16:33 浏览: 44
好的,以下是用 MATLAB 写的 A* 算法示例代码,并加上了中文注释:
```
function [path, cost] = a_star(start, goal, map)
% A* 算法实现
% Inputs:
% start: 出发点坐标 [x,y]
% goal: 目标点坐标 [x,y]
% map: 环境地图,0 表示自由空间,1 表示障碍物
% Outputs:
% path: 从出发点到目标点的路径,以 [x,y] 坐标的列表形式表示
% cost: 路径的代价
% 定义启发式函数:欧几里得距离
heuristic = @(a,b) sqrt((a(1)-b(1))^2 + (a(2)-b(2))^2);
% 定义代价函数
cost_function = @(a,b) ((a(1)-b(1))^2 + (a(2)-b(2))^2);
% 初始化出发点
start_node.g_cost = 0;
start_node.h_cost = heuristic(start, goal);
start_node.f_cost = start_node.g_cost + start_node.h_cost;
start_node.coordinates = start;
start_node.parent = [];
% 初始化开放集合和关闭集合
open_set = [start_node];
closed_set = [];
% 循环直到到达目标点或无法到达
while ~isempty(open_set)
% 找到 f_cost 值最小的节点
current_node_idx = 1;
current_node = open_set(current_node_idx);
for i = 1:length(open_set)
if open_set(i).f_cost < current_node.f_cost
current_node_idx = i;
current_node = open_set(current_node_idx);
end
end
% 检查是否到达目标点
if current_node.coordinates == goal
path = [];
cost = current_node.g_cost;
while ~isempty(current_node.parent)
path = [current_node.coordinates; path];
current_node = current_node.parent;
end
path = [start; path];
return
end
% 从开放集合中移除当前节点并将其添加到关闭集合中
open_set(current_node_idx) = [];
closed_set = [closed_set; current_node];
% 找到当前节点的邻居节点
[neighbors_x, neighbors_y] = find(neighbors(map, current_node.coordinates));
neighbors = [neighbors_x, neighbors_y];
% 循环遍历每个邻居节点
for i = 1:length(neighbors)
neighbor_node.coordinates = neighbors(i,:);
neighbor_node.g_cost = current_node.g_cost + cost_function(current_node.coordinates, neighbor_node.coordinates);
neighbor_node.h_cost = heuristic(neighbor_node.coordinates, goal);
neighbor_node.f_cost = neighbor_node.g_cost + neighbor_node.h_cost;
neighbor_node.parent = current_node;
% 检查邻居节点是否已经在关闭集合中
if any(ismember(closed_set, neighbor_node.coordinates, 'rows'))
continue
end
% 检查邻居节点是否已经在开放集合中
neighbor_idx = find(ismember(open_set, neighbor_node.coordinates, 'rows'));
if ~isempty(neighbor_idx)
% 检查到达邻居节点的新路径是否更优
if neighbor_node.g_cost < open_set(neighbor_idx).g_cost
open_set(neighbor_idx) = neighbor_node;
end
else
% 将邻居节点添加到开放集合中
open_set = [open_set; neighbor_node];
end
end
end
% 如果无法到达目标点
path = [];
cost = inf;
end
function [neighborhood] = neighbors(map, coordinates)
% 返回以给定坐标为中心的 3x3 邻域
neighborhood = map(coordinates(1)-1:coordinates(1)+1, coordinates(2)-1:coordinates(2)+1);
end
```
希望这份代码对你有所帮助!
相关推荐
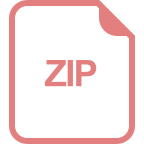
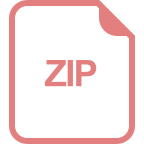
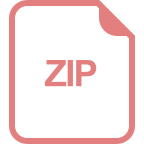














