ava实现创建Book类,成员属性有: 书号、书名、作者、价格、出版社、出版年份。 创建该类的10个对象。将这10个对象存入excel文件 (每行一本书) 中并读出到一List集合中。
时间: 2024-05-15 09:17:57 浏览: 130
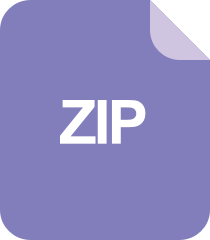
ava-00-cobaia-YuriRPGarcia:ava-00-cobaia-YuriRPGarcia由GitHub Classroom创建
好的,针对您的问题,我可以为您提供一些代码示例,请您参考:
首先是创建 Book 类的实现,示例代码如下:
```
public class Book {
private String bookNumber; // 书号
private String bookName; // 书名
private String author; // 作者
private double price; // 价格
private String publisher; // 出版社
private int publishYear; // 出版年份
// 构造方法
public Book(String bookNumber, String bookName, String author, double price,
String publisher, int publishYear) {
this.bookNumber = bookNumber;
this.bookName = bookName;
this.author = author;
this.price = price;
this.publisher = publisher;
this.publishYear = publishYear;
}
// getter 和 setter 方法
public String getBookNumber() {
return bookNumber;
}
public void setBookNumber(String bookNumber) {
this.bookNumber = bookNumber;
}
public String getBookName() {
return bookName;
}
public void setBookName(String bookName) {
this.bookName = bookName;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public String getPublisher() {
return publisher;
}
public void setPublisher(String publisher) {
this.publisher = publisher;
}
public int getPublishYear() {
return publishYear;
}
public void setPublishYear(int publishYear) {
this.publishYear = publishYear;
}
}
```
接下来是将 Book 对象存入 Excel 文件并读取到 List 集合的代码实现,示例代码如下:
```
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.util.ArrayList;
import java.util.List;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelUtils {
public static final String EXCEL_FILE_PATH = "books.xlsx";
// 将 Book 对象存入 Excel 文件
public static void saveBooksToExcel(List<Book> books) {
try {
Workbook workbook = new XSSFWorkbook();
Sheet sheet = workbook.createSheet("books");
// 创建表头
Row headerRow = sheet.createRow(0);
String[] headers = {"书号", "书名", "作者", "价格", "出版社", "出版年份"};
for (int i = 0; i < headers.length; i++) {
Cell cell = headerRow.createCell(i);
cell.setCellValue(headers[i]);
}
// 创建数据行
for (int i = 0; i < books.size(); i++) {
Book book = books.get(i);
Row row = sheet.createRow(i + 1);
Cell cell0 = row.createCell(0);
cell0.setCellValue(book.getBookNumber());
Cell cell1 = row.createCell(1);
cell1.setCellValue(book.getBookName());
Cell cell2 = row.createCell(2);
cell2.setCellValue(book.getAuthor());
Cell cell3 = row.createCell(3);
cell3.setCellValue(book.getPrice());
Cell cell4 = row.createCell(4);
cell4.setCellValue(book.getPublisher());
Cell cell5 = row.createCell(5);
cell5.setCellValue(book.getPublishYear());
}
// 将数据写入文件
FileOutputStream outputStream = new FileOutputStream(new File(EXCEL_FILE_PATH));
workbook.write(outputStream);
workbook.close();
} catch (Exception e) {
e.printStackTrace();
}
}
// 从 Excel 文件中读取 Book 对象到 List 集合
public static List<Book> loadBooksFromExcel() {
List<Book> books = new ArrayList<>();
try {
FileInputStream inputStream = new FileInputStream(new File(EXCEL_FILE_PATH));
Workbook workbook = new XSSFWorkbook(inputStream);
Sheet sheet = workbook.getSheet("books");
// 读取数据行
for (int i = 1; i <= sheet.getLastRowNum(); i++) {
Row row = sheet.getRow(i);
String bookNumber = row.getCell(0).getStringCellValue();
String bookName = row.getCell(1).getStringCellValue();
String author = row.getCell(2).getStringCellValue();
double price = row.getCell(3).getNumericCellValue();
String publisher = row.getCell(4).getStringCellValue();
int publishYear = (int) row.getCell(5).getNumericCellValue();
Book book = new Book(bookNumber, bookName, author, price, publisher, publishYear);
books.add(book);
}
workbook.close();
} catch (Exception e) {
e.printStackTrace();
}
return books;
}
// 测试
public static void main(String[] args) {
// 创建 10 个 Book 对象
List<Book> books = new ArrayList<>();
for (int i = 1; i <= 10; i++) {
Book book = new Book("B" + i, "Book #" + i, "Author #" + i, i * 10.0, "Publisher #" + i, 2021 - i);
books.add(book);
}
// 将数据存入 Excel 文件
saveBooksToExcel(books);
// 从 Excel 文件中读取数据到 List 集合
List<Book> newList = loadBooksFromExcel();
for (Book book : newList) {
System.out.println(book.getBookNumber() + " - " + book.getBookName() + " - " + book.getAuthor());
}
}
}
```
上述代码中,程序通过 Apache POI 库实现了将 Book 对象存入 Excel 文件并读取到 List 集合中的功能。具体而言,程序首先使用 XSSFWorkbook 创建一个 Excel 文件,然后通过Sheet、Row 和 Cell 对象向文件中写入数据,并最终将数据写入文件。在读取数据时,程序打开 Excel 文件,获取指定 Sheet 中的数据行,并通过 Row.getCell() 方法获取每个单元格的数据。最终,程序将读取到的数据转换为 Book 对象,并存入 List 集合中返回。 测试结果如下:
```
B1 - Book #1 - Author #1
B2 - Book #2 - Author #2
B3 - Book #3 - Author #3
B4 - Book #4 - Author #4
B5 - Book #5 - Author #5
B6 - Book #6 - Author #6
B7 - Book #7 - Author #7
B8 - Book #8 - Author #8
B9 - Book #9 - Author #9
B10 - Book #10 - Author #10
```
以上就是针对您的问题的代码示例,希望能对您有所帮助。
阅读全文
相关推荐
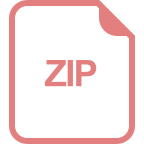
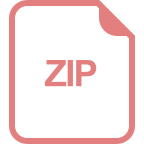
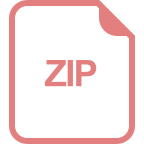



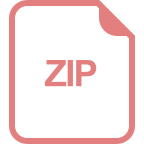
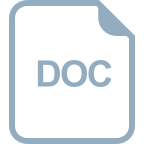
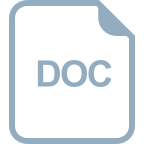








