写一个基于python和opencv的数字图像处理车牌识别代码
时间: 2023-10-11 08:05:58 浏览: 92
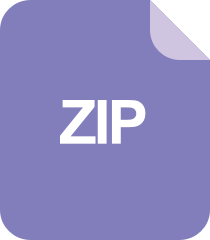
基于Python-opencv的车牌识别

好的,我可以帮你回答这个问题。以下是一个基于 Python 和 OpenCV 的车牌识别代码示例:
1. 导入相关库
```
import cv2
import numpy as np
from PIL import Image
```
2. 读取图片并进行预处理
```
img = cv2.imread('car.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
blur = cv2.GaussianBlur(gray, (5,5), 0)
edges = cv2.Canny(blur, 100, 200)
```
3. 寻找轮廓
```
contours, hierarchy = cv2.findContours(edges.copy(), cv2.RETR_LIST, cv2.CHAIN_APPROX_SIMPLE)
```
4. 筛选可能的车牌区域
```
plates = []
for cnt in contours:
area = cv2.contourArea(cnt)
if area > 1000:
x,y,w,h = cv2.boundingRect(cnt)
aspect_ratio = float(w)/h
if aspect_ratio > 2.0 and aspect_ratio < 5.0:
plates.append(cnt)
```
5. 识别车牌号码
```
for plate in plates:
x,y,w,h = cv2.boundingRect(plate)
roi = gray[y:y+h, x:x+w]
thresh = cv2.threshold(roi, 0, 255, cv2.THRESH_BINARY_INV | cv2.THRESH_OTSU)[1]
kernel = np.ones((3,3), np.uint8)
thresh = cv2.morphologyEx(thresh, cv2.MORPH_OPEN, kernel)
text = pytesseract.image_to_string(Image.fromarray(thresh), lang='eng', config='--psm 7')
print('Detected Plate Number:', text.strip())
```
以上就是一个基本的车牌识别代码示例,供您参考。需要注意的是,该代码需要安装 pytesseract 库,并且需要在计算机中安装 tesseract OCR 引擎才能正常工作。
阅读全文
相关推荐
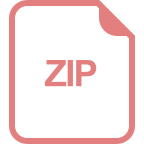
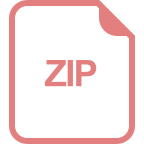
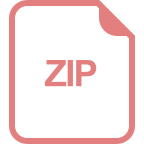
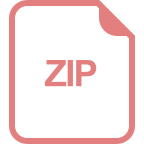
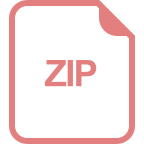
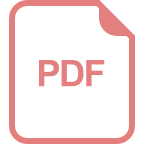
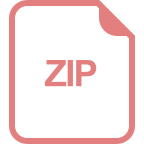
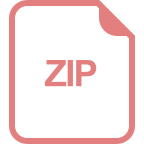
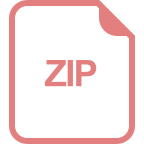
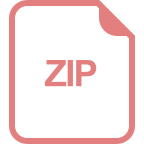
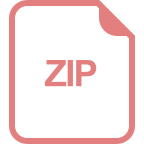
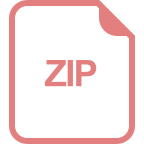
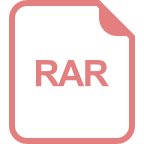
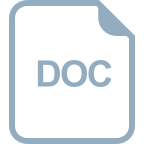
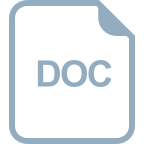
