用vue组件写一个随音乐字节滚动的音乐播放器代码
时间: 2023-08-23 19:20:13 浏览: 90
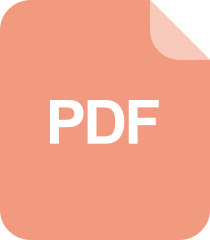
基于vue-element组件实现音乐播放器功能

好的,下面是一个简单的示例代码:
```vue
<template>
<div class="music-player">
<div class="music-info">
<div class="music-title">{{ currentMusic.title }}</div>
<div class="music-artist">{{ currentMusic.artist }}</div>
</div>
<div class="music-progress">
<div class="music-progress-bar" :style="{ width: progressWidth }"></div>
</div>
<div class="music-controls">
<i class="play-btn" :class="{ 'icon-pause': isPlaying, 'icon-play': !isPlaying }" @click="togglePlay"></i>
<i class="prev-btn icon-prev" @click="prevMusic"></i>
<i class="next-btn icon-next" @click="nextMusic"></i>
</div>
</div>
</template>
<script>
export default {
data() {
return {
playlist: [
{
title: '歌曲1',
artist: '歌手1',
url: 'music1.mp3',
duration: 180,
},
{
title: '歌曲2',
artist: '歌手2',
url: 'music2.mp3',
duration: 240,
},
{
title: '歌曲3',
artist: '歌手3',
url: 'music3.mp3',
duration: 200,
},
],
currentMusic: null,
currentIndex: -1,
isPlaying: false,
progressWidth: 0,
timer: null,
};
},
mounted() {
this.playNext();
},
computed: {
currentDuration() {
if (!this.currentMusic) {
return 0;
}
return this.currentMusic.duration;
},
},
methods: {
playNext() {
this.currentIndex++;
if (this.currentIndex >= this.playlist.length) {
this.currentIndex = 0;
}
this.currentMusic = this.playlist[this.currentIndex];
this.playMusic();
},
playPrev() {
this.currentIndex--;
if (this.currentIndex < 0) {
this.currentIndex = this.playlist.length - 1;
}
this.currentMusic = this.playlist[this.currentIndex];
this.playMusic();
},
playMusic() {
this.isPlaying = true;
this.progressWidth = 0;
clearInterval(this.timer);
this.timer = setInterval(() => {
this.progressWidth += 0.1;
if (this.progressWidth >= 100) {
this.playNext();
}
}, this.currentDuration / 1000);
},
togglePlay() {
if (this.isPlaying) {
clearInterval(this.timer);
} else {
this.timer = setInterval(() => {
this.progressWidth += 0.1;
if (this.progressWidth >= 100) {
this.playNext();
}
}, this.currentDuration / 1000);
}
this.isPlaying = !this.isPlaying;
},
prevMusic() {
this.playPrev();
},
nextMusic() {
this.playNext();
},
},
};
</script>
<style>
.music-player {
width: 300px;
height: 100px;
background-color: #f9f9f9;
border: 1px solid #ddd;
border-radius: 4px;
padding: 10px;
display: flex;
align-items: center;
justify-content: space-between;
font-size: 14px;
color: #333;
}
.music-info {
display: flex;
flex-direction: column;
align-items: flex-start;
}
.music-title {
font-size: 16px;
font-weight: bold;
margin-bottom: 5px;
}
.music-progress {
width: 100%;
height: 4px;
background-color: #ddd;
margin: 5px 0;
position: relative;
}
.music-progress-bar {
height: 100%;
background-color: #333;
position: absolute;
top: 0;
left: 0;
}
.music-controls {
display: flex;
align-items: center;
}
.play-btn {
font-size: 20px;
cursor: pointer;
margin-right: 10px;
}
.icon-prev,
.icon-next {
font-size: 20px;
cursor: pointer;
margin-left: 10px;
}
</style>
```
这个示例代码是一个简单的音乐播放器,其中包含了播放/暂停、上一首、下一首等功能,并且可以随着音乐播放进度在页面上滚动字节条。你可以根据自己的需求进行修改和完善。
阅读全文
相关推荐
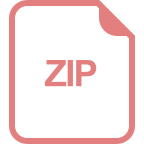
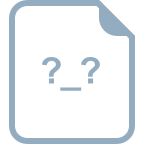
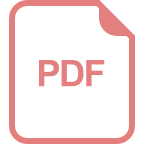
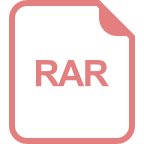
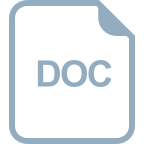
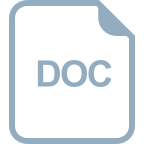
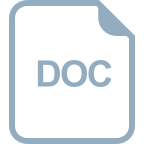
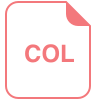
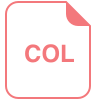
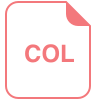
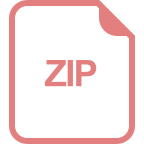
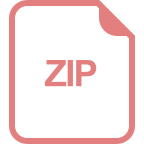
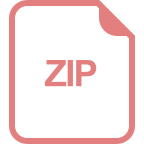