我有一个已经名为“cnl_done.txt”的用空格分好词的文本,要用n元模型再对其进行分词,最后将分词结果输出为一个txt文件,要求这个文件是用“/”分词的,你能给我代码吗
时间: 2024-02-05 15:11:58 浏览: 15
当然可以,以下是一个Python的样例代码,它使用了nltk库来实现n元模型,使用了jieba库来进行中文分词:
```python
import nltk
import jieba
# 读入已分好词的文本
with open('cnl_done.txt', 'r', encoding='utf-8') as f:
text = f.read()
# 将文本按空格分成单词列表
words = text.split()
# 定义n元模型中的n值
n = 2
# 使用nltk库的ngrams函数生成n元组
ngrams = nltk.ngrams(words, n)
# 将n元组转换为字符串形式的词语列表,并使用jieba库进行中文分词
seg_list = []
for gram in ngrams:
seg_list.extend(jieba.cut(''.join(gram)))
# 将分词结果用“/”连接成字符串
seg_result = '/'.join(seg_list)
# 将分词结果输出到文件
with open('seg_result.txt', 'w', encoding='utf-8') as f:
f.write(seg_result)
```
需要注意的是,这个代码中使用了jieba库进行中文分词,所以需要先安装jieba库。可以使用以下命令来安装jieba:
```
pip install jieba
```
相关问题
我有一个已经名为“cnl_done.txt”的用空格分好词的文本,要用n元模型再对其进行分词,你能给我代码吗
当使用n元模型时,我们将文本分成n个词块。然后,我们可以使用这些词块来预测下一个单词。在这个过程中,我们可以使用n-1个词块来预测下一个词块,从而得到一个n元模型。
以下是一个示例代码,使用n元模型对已分好词的文本进行分词:
```python
import re
# 读取已分好词的文本
with open('cnl_done.txt', 'r', encoding='utf-8') as file:
text = file.read()
# 将文本分成单词列表
words = re.findall(r'\w+', text)
# 定义n元模型的n值
n = 3
# 创建n元字典
ngrams = {}
for i in range(len(words)-n+1):
ngram = ' '.join(words[i:i+n-1])
next_word = words[i+n-1]
if ngram not in ngrams.keys():
ngrams[ngram] = []
ngrams[ngram].append(next_word)
# 预测下一个单词
current_ngram = ' '.join(words[:n-1])
for i in range(100):
if current_ngram not in ngrams.keys():
break
possible_words = ngrams[current_ngram]
next_word = possible_words[0]
print(next_word, end=' ')
current_ngram = ' '.join(words[i+1:i+n-1] + [next_word])
```
这段代码创建一个3元模型,并输出接下来的100个预测单词。你可以根据需要调整n值和预测单词的数量。
我有一个已经名为“cnl_done.txt”的用空格分好词的文本,要用n元模型再对其进行分词,最后将分词结果输出为一个txt文件,你能给我代码吗
当然,我可以给你一个Python的代码示例。以下是一个基于nltk库的n元分词器:
```python
import nltk
from nltk.util import ngrams
# 读取已经分好词的文本
with open('cnl_done.txt', 'r', encoding='utf-8') as f:
text = f.read()
# 将文本转换为词语列表
words = text.split()
# 将词语列表转换为n元组列表
n = 2 # 设置n元模型的n值
ngrams_list = list(ngrams(words, n))
# 将n元组列表中的每个元组转换为字符串,并用空格连接
ngrams_str_list = [' '.join(ngram) for ngram in ngrams_list]
# 将结果写入文件
with open('output.txt', 'w', encoding='utf-8') as f:
f.write('\n'.join(ngrams_str_list))
```
该代码将读取名为“cnl_done.txt”的文件,并将其转换为词语列表。然后,该代码将生成n元组列表,并将其转换为字符串列表。最后,该代码将结果写入名为“output.txt”的文件中。你可以根据需要修改参数n的值来指定不同的n元模型的n值。
相关推荐
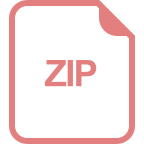







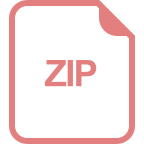
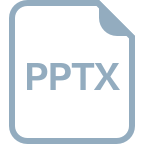
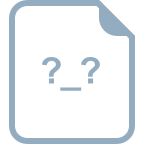