C++实现编写一个程序,统计一个文本文件的行数
时间: 2024-01-22 09:18:22 浏览: 35
可以使用C++的文件流和计数器来实现统计文本文件的行数。以下是一个简单的示例代码:
```cpp
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main()
{
string filename;
cout << "请输入文件名:";
cin >> filename;
ifstream fin(filename);
if (!fin)
{
cerr << "文件打开失败!" << endl;
return 1;
}
int count = 0;
string line;
while (getline(fin, line))
{
++count;
}
cout << "文件" << filename << "共有" << count << "行。" << endl;
fin.close();
return 0;
}
```
该程序首先提示用户输入文件名,然后打开文件并逐行读取文件内容,同时计算行数。最后输出统计结果并关闭文件流。
需要注意的是,在打开文件时需要检查文件是否成功打开,如果打开失败需要及时退出程序并提示用户。此外,程序还需要在操作完成后关闭文件流。
相关问题
用c++编写一个程序,统计一个文本文件的行数,并逐句解释
以下是一个用C++编写的程序,可以统计一个文本文件的行数:
```c++
#include <iostream>
#include <fstream>
#include <string>
int main()
{
// 打开文本文件
std::ifstream file("example.txt");
if (!file.is_open())
{
std::cerr << "Failed to open file!" << std::endl;
return 1;
}
// 统计行数
int count = 0;
std::string line;
while (std::getline(file, line))
{
++count;
}
// 输出结果
std::cout << "The file has " << count << " lines." << std::endl;
return 0;
}
```
首先,我们使用 `ifstream` 类来打开一个文本文件,并检查是否成功打开。如果打开失败,则输出错误信息并退出程序。
接着,我们定义一个计数器 `count`,并使用 `getline` 函数逐行读取文件内容,并将计数器加一。
最后,我们输出计数器的值,即为文件的行数。
需要注意的是,在 `getline` 函数中,每次读取一行文本时,行末的换行符 `\n` 会被自动删除。因此,如果要统计包括空行在内的总行数,需要在计数器加一之前判断当前行是否为空行。
现有一文本文件,需要编写一个c++程序对该文件的行数进行计算(包括空行),并输出。文件每一行不超过80个字符。
可以使用C++的文件输入输出流来实现该程序。具体步骤如下:
1. 打开文件并创建输入流对象。
2. 读取每一行并计数,直到读到文件末尾。
3. 输出计数结果。
以下是示例代码:
```c++
#include <iostream>
#include <fstream>
using namespace std;
int main()
{
string filename;
int count = 0;
cout << "请输入文件名:" << endl;
cin >> filename;
ifstream infile(filename);
if (!infile)
{
cout << "无法打开文件!" << endl;
return 1;
}
string line;
while (getline(infile, line))
{
count++;
}
cout << "文件行数为:" << count << endl;
infile.close();
return 0;
}
```
注:在程序运行完毕后,需要关闭文件流对象。```infile.close();```
相关推荐
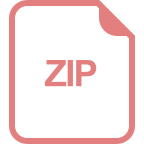
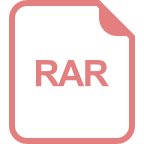
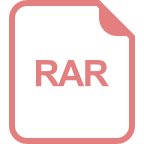








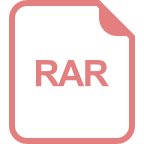
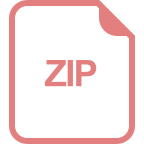
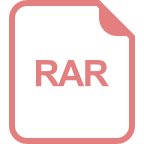
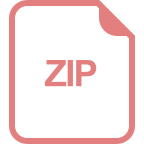
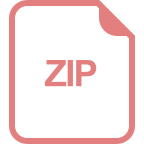