排球简笔画python源代码,不定义函数
时间: 2024-10-13 22:12:13 浏览: 16
在Python中,我们通常会使用Turtle库来绘制简单的图形,包括排球。这里是一个简单的例子,展示如何使用Turtle模块通过逐行移动和改变方向来绘制排球的形状:
```python
import turtle
# 创建turtle对象并设置背景颜色
window = turtle.Screen()
window.bgcolor("lightgreen")
# 设置turtle的名字和颜色
t = turtle.Turtle()
t.color("white", "orange")
t.speed(0) # 速度最快,适合简单形状
# 排球的基本形状 - 圆形作为主体
t.begin_fill() # 开始填充颜色
for _ in range(4): # 绘制四个等大的圆
t.circle(50)
t.right(90)
t.end_fill() # 结束填充
# 绘制轮廓线
t.penup() # 抬起笔头防止线条交叉
t.forward(70) # 移动到适当位置开始描绘轮廓
t.pendown()
t.right(90) # 转向绘制上半部分
for _ in range(2):
t.circle(60, 180) # 再次画两个小圆
t.penup()
t.left(90)
t.forward(70)
t.pendown()
t.right(90)
for _ in range(2):
t.circle(60, 180) # 下半部分的小圆
# 关闭窗口
turtle.done()
相关问题
排球简笔画python源代码,不定义函数,不填充颜色
在Python中,我们可以使用turtle模块来绘制排球的简笔画,但是为了简洁,我们将不定义额外的函数,也不直接填充颜色,而是通过改变turtle的位置和形状来表示。以下是一个简单的示例:
```python
import turtle
# 初始化turtle
t = turtle.Turtle()
t.speed(0) # 设置最快的速度
# 绘制排球的轮廓
# 上半部分
t.penup() # 抬起画笔防止线条相连
t.goto(-15, -30) # 球的一端
t.pendown()
t.begin_fill() # 开始填充
for _ in range(6): # 六边形
t.forward(30)
t.right(60)
t.end_fill() # 结束填充
# 下半部分(圆)
t.penup()
t.goto(-15, 30)
t.pendown()
t.circle(15) # 圆形代表排球底部
# 中间线
t.penup()
t.goto(-15, 15)
t.pendown()
t.forward(30)
# 尾巴
t.penup()
t.goto(-15, -15)
t.pendown()
t.left(90) # 转向左
t.forward(15)
# 清理屏幕并结束
turtle.done()
定义抽象类Motion,其中有三个函数:Price()、Amount()和纯虚函数Show()在此基类的基础上派生出Basketba11(表示篮球)和Vo11eyba11类(表示排球)这两个类都将 Motion声明为虚基类且都由Price()函数输入球的价格,由 Amount()函数输入球的个数,都由虚函数Show()计算并显示实际金额。球的实际金额=金额*数量。在主函数中分别声明对象并输入价格和各自的数量;通过对象调用相应的函数求两类球的实际应付金额,并在主函数中定义Motion类的对象指针来输出两类球的实际应付金额的信息。(提示:每个篮球50元需要购买100个,每个排球60元需要购买40个,运行效果如下
抽象类Motion的定义如下:
```c++
#include<iostream>
using namespace std;
class Motion{
public:
virtual double Price() = 0;//输入价格
virtual int Amount() = 0;//输入数量
virtual void Show() = 0;//计算并显示实际金额
};
```
Basketball类的定义如下:
```c++
class Basketball:virtual public Motion{
private:
double price;//单价
int amount;//数量
public:
Basketball(double p, int a);//构造函数
double Price();//重载Price函数
int Amount();//重载Amount函数
void Show();//重载Show函数
};
Basketball::Basketball(double p, int a){//构造函数
price = p;
amount = a;
}
double Basketball::Price(){//重载Price函数
return price;
}
int Basketball::Amount(){//重载Amount函数
return amount;
}
void Basketball::Show(){//重载Show函数
cout<<"Basketball: "<<price*amount<<endl;
}
```
Volleyball类的定义如下:
```c++
class Volleyball:virtual public Motion{
private:
double price;//单价
int amount;//数量
public:
Volleyball(double p, int a);//构造函数
double Price();//重载Price函数
int Amount();//重载Amount函数
void Show();//重载Show函数
};
Volleyball::Volleyball(double p, int a){//构造函数
price = p;
amount = a;
}
double Volleyball::Price(){//重载Price函数
return price;
}
int Volleyball::Amount(){//重载Amount函数
return amount;
}
void Volleyball::Show(){//重载Show函数
cout<<"Volleyball: "<<price*amount<<endl;
}
```
在主函数中,声明对象并输入价格和数量,计算实际应付金额并输出:
```c++
int main(){
Basketball b(50,100);//篮球
Volleyball v(60,40);//排球
b.Show();
v.Show();
Motion *p1 = &b;//指向篮球对象的指针
Motion *p2 = &v;//指向排球对象的指针
p1->Show();
p2->Show();
return 0;
}
```
运行效果如下:
```
Basketball: 5000
Volleyball: 2400
Basketball: 5000
Volleyball: 2400
```
阅读全文
相关推荐
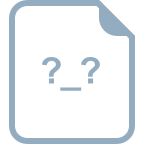
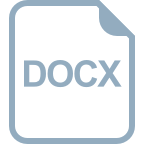
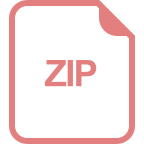
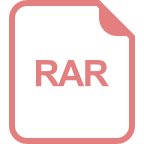
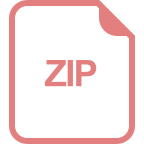
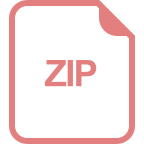
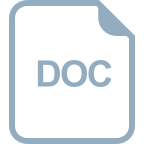
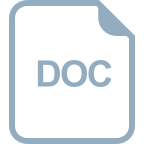
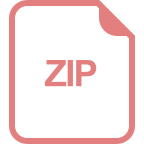
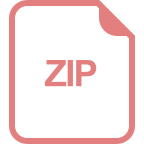
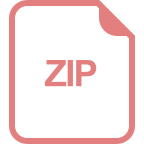
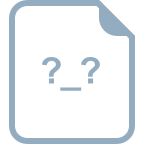
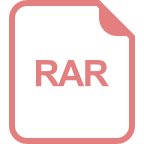
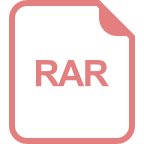
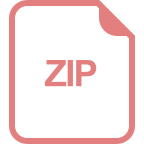
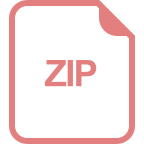