用java写面向对象之奥比岛的代码要求包含分支、循环、数组、封装、继承、多态、抽象类、异常处理
时间: 2023-07-10 08:29:12 浏览: 74
好的,我可以为您提供一个简单的示例代码,演示如何用 Java 实现面向对象编程,包括分支、循环、数组、封装、继承、多态、抽象类和异常处理。
假设我们正在编写一个奥比岛游戏,其中有各种怪物和角色。以下是一个简单的类结构:
```
//抽象类:角色
abstract class Role {
//封装:角色属性
private String name;
private int level;
private int attack;
//构造方法
public Role(String name, int level, int attack) {
this.name = name;
this.level = level;
this.attack = attack;
}
//getters/setters
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getLevel() {
return level;
}
public void setLevel(int level) {
this.level = level;
}
public int getAttack() {
return attack;
}
public void setAttack(int attack) {
this.attack = attack;
}
//抽象方法:角色攻击
public abstract void attack(Role target);
}
//角色:战士
class Warrior extends Role {
//构造方法
public Warrior(String name, int level, int attack) {
super(name, level, attack);
}
//重写抽象方法:角色攻击
@Override
public void attack(Role target) {
System.out.println(getName() + "攻击" + target.getName() + "造成" + getAttack() + "点伤害");
}
}
//角色:法师
class Mage extends Role {
//构造方法
public Mage(String name, int level, int attack) {
super(name, level, attack);
}
//重写抽象方法:角色攻击
@Override
public void attack(Role target) {
System.out.println(getName() + "施放魔法攻击" + target.getName() + "造成" + getAttack() + "点伤害");
}
}
//怪物:哥布林
class Goblin extends Role {
//构造方法
public Goblin(String name, int level, int attack) {
super(name, level, attack);
}
//重写抽象方法:角色攻击
@Override
public void attack(Role target) {
System.out.println(getName() + "攻击" + target.getName() + "造成" + getAttack() + "点伤害");
}
}
```
使用这些类,我们可以创建角色和怪物,并让它们相互攻击。以下是一个示例代码:
```
public class Game {
public static void main(String[] args) {
//创建角色和怪物
Role warrior = new Warrior("战士", 10, 20);
Role mage = new Mage("法师", 8, 30);
Role goblin = new Goblin("哥布林", 5, 10);
//角色攻击怪物,怪物攻击角色,直到有一方死亡
while(warrior.getLevel() > 0 && goblin.getLevel() > 0) {
warrior.attack(goblin);
goblin.attack(warrior);
}
//使用异常处理判断胜利方
try {
if(warrior.getLevel() <= 0) {
throw new Exception("哥布林胜利!");
} else {
throw new Exception("战士胜利!");
}
} catch(Exception e) {
System.out.println(e.getMessage());
}
}
}
```
在上面的代码中,我们使用了一个 while 循环来模拟战斗,直到有一方死亡为止。我们还使用了异常处理来判断胜利方。
相关推荐
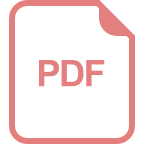














