c++实现DES算法在CBC模式下】
时间: 2023-11-30 13:24:44 浏览: 82
好的,下面是C++实现DES算法在CBC模式下的代码:
```cpp
#include <iostream>
#include <cstring>
#include <openssl/des.h>
using namespace std;
void des_cbc_encrypt(const unsigned char *input, unsigned char *output, const unsigned char *key, const unsigned char *iv, int length) {
DES_cblock key_encrypt;
DES_key_schedule schedule;
memcpy(key_encrypt, key, 8);
DES_set_key_checked(&key_encrypt, &schedule);
DES_cblock iv_encrypt;
memcpy(iv_encrypt, iv, 8);
DES_ncbc_encrypt(input, output, length, &schedule, &iv_encrypt, DES_ENCRYPT);
}
void des_cbc_decrypt(const unsigned char *input, unsigned char *output, const unsigned char *key, const unsigned char *iv, int length) {
DES_cblock key_encrypt;
DES_key_schedule schedule;
memcpy(key_encrypt, key, 8);
DES_set_key_checked(&key_encrypt, &schedule);
DES_cblock iv_encrypt;
memcpy(iv_encrypt, iv, 8);
DES_ncbc_encrypt(input, output, length, &schedule, &iv_encrypt, DES_DECRYPT);
}
int main() {
unsigned char key[] = "12345678"; // 8字节密钥
unsigned char iv[] = "abcdefgh"; // 8字节IV
unsigned char plaintext[] = "Hello World!";
unsigned char ciphertext[1024] = {0};
unsigned char decrypted[1024] = {0};
int plaintext_len = strlen((char *)plaintext);
int ciphertext_len = plaintext_len + 8 - plaintext_len % 8;
des_cbc_encrypt(plaintext, ciphertext, key, iv, ciphertext_len);
cout << "Encrypted Text: " << ciphertext << endl;
des_cbc_decrypt(ciphertext, decrypted, key, iv, ciphertext_len);
cout << "Decrypted Text: " << decrypted << endl;
return 0;
}
```
在这个例子中,我们使用了OpenSSL库中的DES实现来进行加密和解密。首先,我们定义了`des_cbc_encrypt`和`des_cbc_decrypt`函数来分别进行加密和解密操作。其中,`input`参数是输入的明文或密文,`output`参数是输出的密文或明文,`key`参数是密钥,`iv`参数是初始化向量,`length`参数是输入的数据长度。在加密和解密时,我们首先需要将密钥和IV转换成DES_cblock类型,然后使用`DES_set_key_checked`函数来生成密钥的调度表。接下来,我们使用`DES_ncbc_encrypt`函数来进行加密或解密操作。最后,我们使用`cout`输出加密后的密文和解密后的明文。
注意,这里我们假设输入的数据长度是8的倍数,如果不是8的倍数,则需要在末尾填充一些数据,使其长度变为8的倍数。这里我们使用的是补0的方式,在实际使用时需要根据需求选择合适的填充方式。
阅读全文
相关推荐
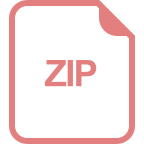

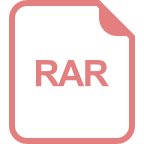
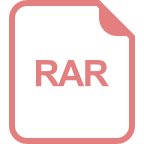
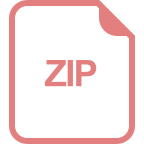
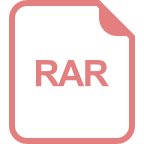
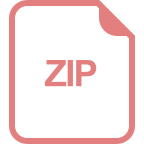
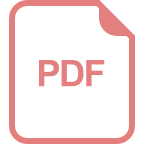
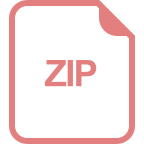
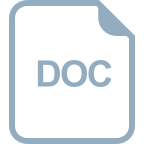
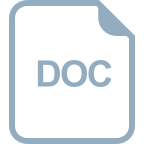
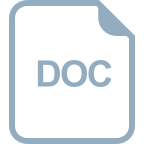
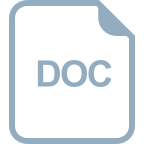
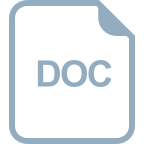

