利用文件IO知识获取music目录下的所有mp3文件名和picture目录下所有bmp、jpg、jpeg文件名,把获取到的文件名作为数据域存入链表(链表类型自选); 显示出功能选择界面:音乐播放、图片浏览; 音乐播放功能: (1)支持播放mp3格式音乐; (2)通过触摸屏点击,支持上一曲、下一曲、播放、暂停、结束播放、返回功能选择界面; (3)音乐播放期间,可以返回功能选择界面,进入图片浏览界面。 图片浏览功能: (1)支持显示bmp、jpg、jpeg格式图片; (2)支持自动播放模式、手动浏览模式切换;自动播放每隔3S换下一幅图;手动浏览通过触摸屏点击,实现上一幅、下一幅切换;5S没有点击上一幅或下一幅,自动切换到自动播放模式; (3)有返回按钮,能否返回功能选择界面。
时间: 2023-12-23 20:05:41 浏览: 103
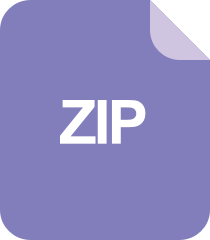
获取指定目录下的文件列表 获取指定目录下的所有文件名和路径(包括子目录)

好的,我可以为您提供一个Python的代码框架,实现上述功能。
```python
import os
import pygame
from PIL import Image
from collections import deque
# 获取音乐和图片文件名
music_path = "music"
picture_path = "picture"
music_files = [f for f in os.listdir(music_path) if f.endswith(".mp3")]
picture_files = [f for f in os.listdir(picture_path) if f.endswith(".bmp") or f.endswith(".jpg") or f.endswith(".jpeg")]
# 将文件名作为数据域存入链表
music_list = deque(music_files)
picture_list = deque(picture_files)
# 初始化pygame
pygame.init()
# 创建窗口
window = pygame.display.set_mode((800, 600))
# 加载音乐
pygame.mixer.music.load(os.path.join(music_path, music_list[0]))
# 加载图片
cur_picture = Image.open(os.path.join(picture_path, picture_list[0]))
# 设置自动播放和手动浏览模式
auto_play = True
play_time = 0
# 显示功能选择界面
def show_menu():
# TODO: 在窗口中显示功能选择界面
pass
# 播放音乐
def play_music():
global music_list
global auto_play
global play_time
# 播放音乐
pygame.mixer.music.play()
# 循环监听事件
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
# 上一曲
music_list.rotate(1)
pygame.mixer.music.load(os.path.join(music_path, music_list[0]))
pygame.mixer.music.play()
elif event.key == pygame.K_RIGHT:
# 下一曲
music_list.rotate(-1)
pygame.mixer.music.load(os.path.join(music_path, music_list[0]))
pygame.mixer.music.play()
elif event.key == pygame.K_SPACE:
# 播放/暂停
if pygame.mixer.music.get_busy():
pygame.mixer.music.pause()
else:
pygame.mixer.music.unpause()
elif event.key == pygame.K_ESCAPE:
# 结束播放
pygame.mixer.music.stop()
return
elif event.key == pygame.K_RETURN:
# 返回功能选择界面
pygame.mixer.music.stop()
show_menu()
return
# 自动播放模式
if auto_play:
play_time += 1
if play_time >= 3000:
play_time = 0
music_list.rotate(-1)
pygame.mixer.music.load(os.path.join(music_path, music_list[0]))
pygame.mixer.music.play()
else:
play_time = 0
# 浏览图片
def browse_picture():
global picture_list
global auto_play
global play_time
global cur_picture
# 循环监听事件
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
# 上一幅
picture_list.rotate(1)
cur_picture = Image.open(os.path.join(picture_path, picture_list[0]))
auto_play = False
elif event.key == pygame.K_RIGHT:
# 下一幅
picture_list.rotate(-1)
cur_picture = Image.open(os.path.join(picture_path, picture_list[0]))
auto_play = False
elif event.key == pygame.K_ESCAPE:
# 返回功能选择界面
auto_play = True
show_menu()
return
# 自动播放模式
if auto_play:
play_time += 1
if play_time >= 3000:
play_time = 0
picture_list.rotate(-1)
cur_picture = Image.open(os.path.join(picture_path, picture_list[0]))
else:
play_time = 0
# 显示当前图片
cur_picture.show()
# 显示功能选择界面
show_menu()
```
此代码框架中,我们首先利用文件IO知识获取了music目录下的所有mp3文件名和picture目录下所有bmp、jpg、jpeg文件名,并将它们作为数据域存入双向链表中。然后,我们使用pygame和PIL等库来实现音乐播放和图片浏览等功能,并在程序中实现了自动播放和手动浏览模式的切换,以及返回功能选择界面的方法。希望这个代码框架能够对您有所帮助。
阅读全文
相关推荐
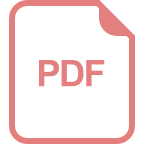
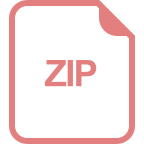
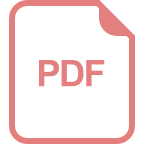
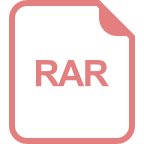
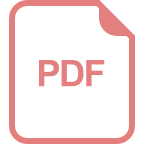
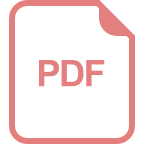
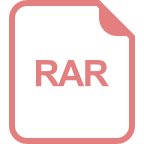
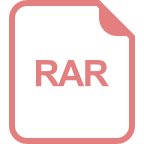
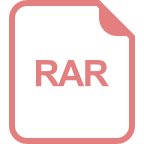
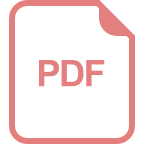
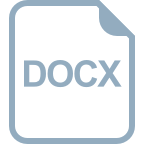
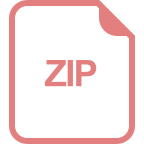
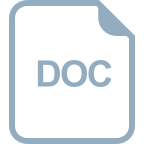
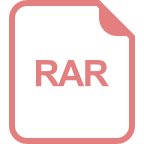
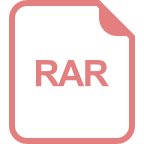
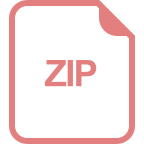
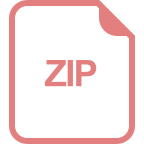
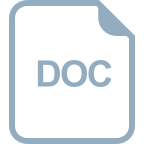
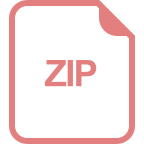