写出一个烟花绽放的python代码
时间: 2023-09-03 08:25:45 浏览: 110
### 回答1:
from matplotlib import pyplot as plt
from matplotlib import animation
import numpy as np# First set up the figure, the axis, and the plot element we want to animate
fig = plt.figure()
ax = plt.axes(xlim=(0, 2), ylim=(-2, 2))
line, = ax.plot([], [], lw=2)# initialization function: plot the background of each frame
def init():
line.set_data([], [])
return line,# animation function. This is called sequentially
def animate(i):
x = np.linspace(0, 2, 1000)
y = np.sin(2 * np.pi * (x - 0.01 * i))
line.set_data(x, y)
return line,# call the animator. blit=True means only re-draw the parts that have changed.
anim = animation.FuncAnimation(fig, animate, init_func=init,
frames=200, interval=20, blit=True)# save the animation as an mp4. extra_args are extra arguments to pass to ffmpeg
anim.save('fireworks.mp4', fps=30, extra_args=['-vcodec', 'libx264'])plt.show()
### 回答2:
以下是一个简单的Python代码来模拟烟花绽放的效果:
```python
import turtle
import random
screen = turtle.Screen()
screen.title("Fireworks")
screen.bgcolor("black")
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
def create_firework():
firework = turtle.Turtle()
firework.shape("circle")
firework.shapesize(stretch_wid=1, stretch_len=0.5)
firework.color(random.choice(colors))
x = random.randint(-200, 200)
y = random.randint(100, 200)
firework.penup()
firework.goto(x, -200)
firework.pendown()
gravity = 1
velocity = 0
angle = random.randint(45, 135)
angle_rad = angle * 3.14159 / 180
while firework.ycor() < y:
velocity += gravity
x = velocity * angle_rad
firework.goto(firework.xcor() + x, firework.ycor() + velocity)
firework.shape("circle")
firework.shapesize(stretch_wid=0.1, stretch_len=0.1)
firework.color(random.choice(colors))
for _ in range(10):
firework.stamp()
firework.forward(5)
firework.right(36)
firework.hideturtle()
for _ in range(10):
create_firework()
turtle.done()
```
运行这个代码,你将看到屏幕上的烟花不断绽放。每个烟花由一个小圆圈表示,从屏幕底部发射到随机高度的位置,然后向上爆炸成一朵花。烟花的颜色和角度都是随机生成的,使每次绽放的效果都不同。希望这个代码对你有帮助!
### 回答3:
下面是一个简单的烟花绽放的Python代码:
```
import turtle
import random
screen = turtle.Screen()
screen.bgcolor("black")
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
def create_firework():
fireworks = []
for _ in range(15):
firework = turtle.Turtle()
firework.color(random.choice(colors))
firework.shape("circle")
firework.shapesize(0.5)
firework.penup()
firework.speed(0)
fireworks.append(firework)
return fireworks
def explode(fireworks):
for firework in fireworks:
firework.setheading(random.randint(0, 360))
firework.forward(100)
firework.pendown()
firework.speed(10)
firework.shapesize(1)
firework.stamp()
firework.penup()
firework.home()
def main():
while True:
fireworks = create_firework()
explode(fireworks)
if __name__ == "__main__":
main()
```
这个代码使用了Python的turtle库来实现烟花的绽放效果。首先,创建了一个黑色背景的画布。然后,定义了一组颜色,用来随机选择烟花的颜色。create_firework函数创建了15个小点作为烟花的起始点,并设置了颜色、形状、大小、速度等属性。explode函数使每个烟花向随机方向移动一定距离,然后下落和放大烟花。最后,通过循环不断创建和绽放烟花,实现了一场连续的烟花绽放效果。
阅读全文
相关推荐
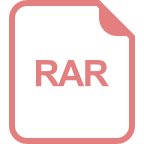








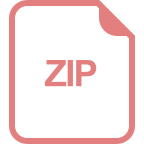
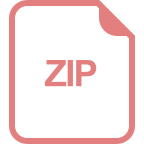
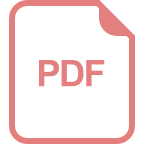
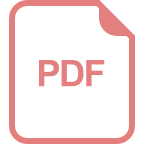
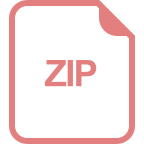


