写一个共享内存用于进程间通信
时间: 2023-12-13 10:02:54 浏览: 81
在Linux下,我们可以使用shmget函数创建一个共享内存区域,使用shmat函数将其映射到进程的地址空间中,然后就可以在不同进程之间共享这块内存了。
下面是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/ipc.h>
#include <sys/shm.h>
int main()
{
int shmid;
char *shmaddr;
key_t key;
// 创建共享内存
key = ftok(".", 's');
if ((shmid = shmget(key, 1024, IPC_CREAT | 0666)) == -1)
{
perror("shmget");
exit(EXIT_FAILURE);
}
// 将共享内存映射到进程地址空间
if ((shmaddr = (char*)shmat(shmid, NULL, 0)) == (char*)-1)
{
perror("shmat");
exit(EXIT_FAILURE);
}
// 写入共享内存
sprintf(shmaddr, "Hello, shared memory!");
// 等待另一个进程读取共享内存内容
sleep(5);
// 打印共享内存内容
printf("Shared memory: %s\n", shmaddr);
// 分离共享内存
if (shmdt(shmaddr) == -1)
{
perror("shmdt");
exit(EXIT_FAILURE);
}
// 删除共享内存
if (shmctl(shmid, IPC_RMID, NULL) == -1)
{
perror("shmctl IPC_RMID");
exit(EXIT_FAILURE);
}
return 0;
}
```
这个程序创建了一个大小为1024字节的共享内存,将其映射到进程地址空间中,写入了一些数据,等待5秒钟后再打印出来,最后分离共享内存并删除它。如果要在另一个进程中读取这个共享内存中的数据,只需要使用相同的key调用shmget和shmat函数即可。
阅读全文
相关推荐
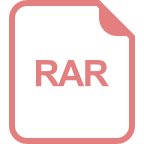











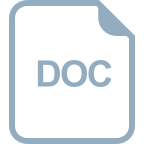

