vue前端如何实现与node.js后端服务器建立连接,给出相应代码。
时间: 2024-03-25 22:40:03 浏览: 70
好的,下面是使用 Vue.js 前端框架与 Node.js 后端建立连接的示例代码。
首先,在 Vue.js 项目中安装 `axios` 模块,它可以用来向后端发送 HTTP 请求:
```
npm install axios --save
```
然后在前端代码中引入 `axios` 模块:
```javascript
import axios from 'axios';
```
接下来,可以使用 `axios` 发送 HTTP 请求与后端建立连接。例如,可以在 Vue 组件中使用 `axios` 发送 GET 请求:
```javascript
axios.get('/api/data')
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error);
});
```
这里假设后端服务器的 API 路径为 `/api/data`,服务器将返回一些数据。当客户端请求该路径时,`axios` 将向后端发送 GET 请求,并在响应成功后将数据打印到控制台中。
当然,你也可以使用 `axios` 发送 POST 请求、PUT 请求等等,具体使用方式可以参考 `axios` 文档或相关教程。
在后端代码中,你需要通过 Node.js 的 `http` 模块或者 `Express` 框架等来接收客户端的请求并处理。例如,可以通过 `http` 模块创建一个简单的服务器:
```javascript
const http = require('http');
const server = http.createServer((req, res) => {
if (req.url === '/api/data') {
res.writeHead(200, {'Content-Type': 'application/json'});
res.write(JSON.stringify({data: 'Hello, World!'}));
res.end();
} else {
res.writeHead(404, {'Content-Type': 'text/plain'});
res.write('404 Not Found');
res.end();
}
});
server.listen(3000, () => {
console.log('Server is running on port 3000.');
});
```
这里假设客户端请求的 API 路径为 `/api/data`,当请求该路径时,服务器将返回一个 JSON 格式的数据 `{data: 'Hello, World!'}`。当请求其他路径时,服务器将返回 404 错误。
当然,你也可以使用 `Express` 框架等来编写后端代码。具体使用方式可以参考相关文档或教程。
希望这些代码能够帮助到你。
阅读全文
相关推荐
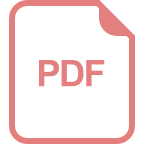
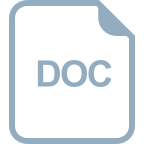
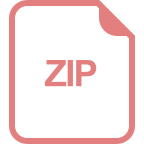
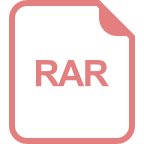
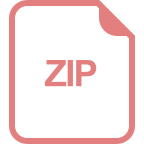
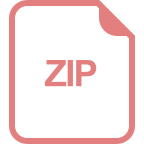
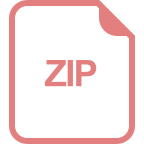
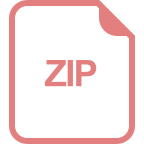
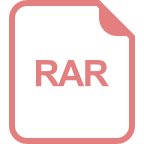
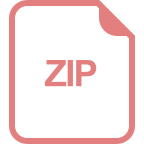
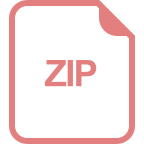
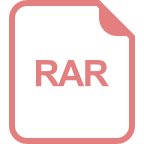
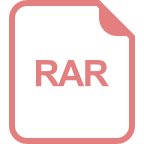
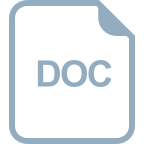
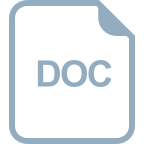
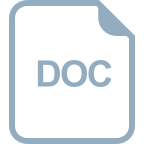
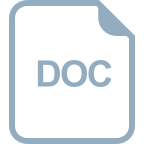