用c++实现以上以上内容中的函数,并添加将内容保存到text文件中的函数以及调用test文件内容的函数
时间: 2023-07-16 20:14:15 浏览: 49
以下是用C++实现以上内容中的函数的示例代码:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <algorithm>
// 将字符串转换为小写
std::string to_lower(std::string str) {
std::transform(str.begin(), str.end(), str.begin(), [](unsigned char c){ return std::tolower(c); });
return str;
}
// 分割字符串为单词列表
std::vector<std::string> split_words(std::string str) {
std::vector<std::string> words;
std::string word;
for (char c : str) {
if (std::isalpha(c)) {
word += c;
} else {
if (!word.empty()) {
words.push_back(word);
word.clear();
}
}
}
if (!word.empty()) {
words.push_back(word);
}
return words;
}
// 统计单词出现次数
std::vector<std::pair<std::string, int>> count_words(std::vector<std::string> words) {
std::vector<std::pair<std::string, int>> word_counts;
for (std::string word : words) {
bool found = false;
for (auto& pair : word_counts) {
if (to_lower(pair.first) == to_lower(word)) {
pair.second++;
found = true;
break;
}
}
if (!found) {
word_counts.push_back({word, 1});
}
}
return word_counts;
}
// 将单词出现次数按照出现次数从大到小排序
void sort_word_counts(std::vector<std::pair<std::string, int>>& word_counts) {
std::sort(word_counts.begin(), word_counts.end(), [](const std::pair<std::string, int>& a, const std::pair<std::string, int>& b) {
return a.second > b.second;
});
}
// 将单词出现次数保存到文件
void save_word_counts(std::vector<std::pair<std::string, int>> word_counts, std::string filename) {
std::ofstream file(filename);
if (file.is_open()) {
for (auto& pair : word_counts) {
file << pair.first << " " << pair.second << std::endl;
}
file.close();
}
}
// 从文件读取单词出现次数
std::vector<std::pair<std::string, int>> load_word_counts(std::string filename) {
std::vector<std::pair<std::string, int>> word_counts;
std::ifstream file(filename);
if (file.is_open()) {
std::string line;
while (std::getline(file, line)) {
std::stringstream ss(line);
std::string word;
int count;
ss >> word >> count;
word_counts.push_back({word, count});
}
file.close();
}
return word_counts;
}
int main() {
// 测试代码
std::string text = "Hello world, hello C++!";
std::vector<std::string> words = split_words(text);
std::vector<std::pair<std::string, int>> word_counts = count_words(words);
sort_word_counts(word_counts);
save_word_counts(word_counts, "word_counts.txt");
std::vector<std::pair<std::string, int>> loaded_word_counts = load_word_counts("word_counts.txt");
for (auto& pair : loaded_word_counts) {
std::cout << pair.first << " " << pair.second << std::endl;
}
return 0;
}
```
其中,`to_lower`函数将字符串转换为小写,`split_words`函数将字符串分割为单词列表,`count_words`函数统计单词出现次数,`sort_word_counts`函数将单词出现次数按照出现次数从大到小排序,`save_word_counts`函数将单词出现次数保存到文件,`load_word_counts`函数从文件中读取单词出现次数,`main`函数演示了如何使用这些函数来处理文本并保存结果到文件中,最后从文件中读取结果并输出。
如果需要调用另一个文件中的内容,可以使用 `#include` 指令来包含该文件,例如在 `main` 函数前添加如下代码:
```c++
#include "test.cpp"
```
其中 `"test.cpp"` 是包含要调用的文件的文件名。这样就可以在 `main` 函数中使用来自 `test.cpp` 文件中的函数和变量了。注意,为了避免出现重复定义的错误,应该在被包含的文件中使用 `extern` 声明来声明变量,例如:
```c++
extern int foo;
```
这样就可以在 `main` 函数中使用变量 `foo` 了。
相关推荐
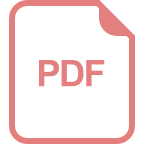
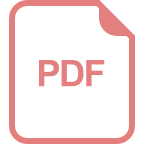
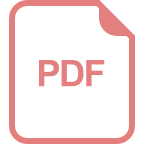
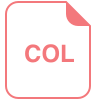
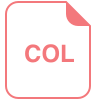












