基于stm32单片机的74hc595驱动8个led灯代码
时间: 2024-02-24 11:54:16 浏览: 98
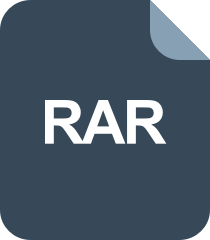
74hc595驱动led8*8
以下是基于STM32单片机的74HC595驱动8个LED灯的代码:
```c
#include "stm32f10x.h"
#define HC595_PORT GPIOA
#define HC595_DS_PIN GPIO_Pin_0
#define HC595_SHCP_PIN GPIO_Pin_1
#define HC595_STCP_PIN GPIO_Pin_2
void HC595_Init(void);
void HC595_Write(uint8_t dat);
int main(void)
{
HC595_Init();
uint8_t led_data = 0x01;
while (1)
{
HC595_Write(led_data);
led_data = (led_data << 1) | (led_data >> 7); //循环移位
for (uint16_t i = 0; i < 10000; i++); //延时
}
}
void HC595_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = HC595_DS_PIN | HC595_SHCP_PIN | HC595_STCP_PIN;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_Init(HC595_PORT, &GPIO_InitStructure);
GPIO_ResetBits(HC595_PORT, HC595_DS_PIN | HC595_SHCP_PIN | HC595_STCP_PIN);
}
void HC595_Write(uint8_t dat)
{
for (uint8_t i = 0; i < 8; i++)
{
if (dat & 0x80) GPIO_SetBits(HC595_PORT, HC595_DS_PIN);
else GPIO_ResetBits(HC595_PORT, HC595_DS_PIN);
GPIO_SetBits(HC595_PORT, HC595_SHCP_PIN);
GPIO_ResetBits(HC595_PORT, HC595_SHCP_PIN);
dat <<= 1;
}
GPIO_SetBits(HC595_PORT, HC595_STCP_PIN);
GPIO_ResetBits(HC595_PORT, HC595_STCP_PIN);
}
```
在此代码中,我们使用了STM32的GPIO模块控制74HC595芯片,来实现控制8个LED灯的功能。具体实现方式为,通过循环移位来得到要显示的数据,并将数据写入到74HC595芯片中,然后通过锁存时钟信号(STCP)将数据传给输出寄存器,最后通过移位时钟信号(SHCP)将输出寄存器中的数据传给移位寄存器,然后通过数据信号(DS)将数据传入到移位寄存器中,完成LED灯的控制。
阅读全文
相关推荐

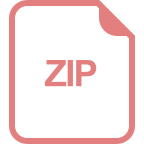
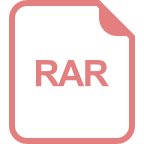
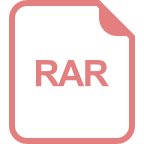


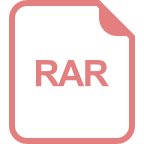
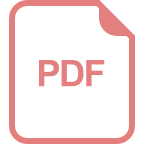
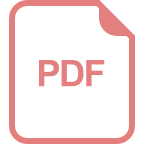
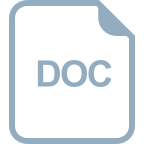
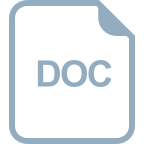

