读取 stu.txt,文件中每行为某同学各科目的成绩,请利用 numpy 中的统计函数进行以下统计:求出每位同学的平均成绩;求出每个科目的最高分与最低分。
时间: 2023-05-02 09:04:34 浏览: 155
这道题目要求读取 stu.txt 文件,每行代表某同学各科目的成绩;请利用 numpy 中的统计函数进行以下统计:求出每位同学的平均成绩;求出每个科目的平均成绩、最高分与最低分。
思路:先使用 numpy 的 loadtxt 函数读取数据,然后使用 axis 参数对数据进行分行或分列处理,最后调用 mean、amax、amin 等函数进行统计计算。
具体实现就这样(假设文件中每行有 4 个数,表示各科成绩):
import numpy as np
# 读取文件,解析为二维数组
data = np.loadtxt('stu.txt')
# 求出每位同学的平均成绩
stu_mean = np.mean(data, axis=1)
print('每位同学的平均成绩:', stu_mean)
# 求出每个科目的平均成绩、最高分与最低分
sub_mean = np.mean(data, axis=0)
sub_max = np.amax(data, axis=0)
sub_min = np.amin(data, axis=0)
print('每个科目的平均成绩:', sub_mean)
print('每个科目的最高分:', sub_max)
print('每个科目的最低分:', sub_min)
注:这里假定所有数据都是数值类型,否则可能需要针对具体情况进行类型转换或处理,比如去除非数值字符。
相关问题
求读取文件stu_score.xlsx,求班级每科成绩的均值、中位数、众数、中列数、极差
在Python中,我们可以使用pandas库来处理Excel文件并计算各类统计指标。假设`stu_score.xlsx`是一个包含学生姓名、班级、科目和分数的数据表,首先需要加载数据:
```python
import pandas as pd
# 加载Excel文件
df = pd.read_excel('stu_score.xlsx')
# 需要确认表格结构,假设'班级'在DataFrame的index列,'科目'和'分数'分别对应其他列
# 假设每个学生的分数在一个单独的列里,如'Score_1', 'Score_2', ..., 'Score_n'
# 计算每班每科的成绩统计信息
class_scores = df.groupby(['班级', '科目']).agg({
'分数': ['mean'] # 平均值 (mean)
, '分数': ['median'] # 中位数 (median)
, '分数': ['mode'] # 众数 (mode) 可能会返回多个值
, '分数': lambda x: len(x) / 2 if len(x) % 2 == 0 else int(len(x) // 2 + 0.5) # 中位数位置 (Q2) 或中列数
, '分数': 'max' - 'min' # 极差 (range)
})
# 现在class_scores DataFrame将包含每个班级每科的成绩统计结果
```
注意:以上代码假设了数据格式,如果实际文件结构不同,你需要调整列名。另外,对于众数(mode),如果某科目有多组数据相同,则可能会返回一组或多组结果。
用python生成一段代码读取txt文件(文件包括学号,姓名,成绩),统计出每个人成绩最高的三次分数,代码用模块函数实现
可以使用Python内置的csv模块来读取包含学号、姓名和成绩的txt文件,然后使用字典来存储每个学生的成绩,最后找到每个学生的最高三次分数。以下是实现代码:
```python
import csv
def read_file(file_name):
# 读取txt文件
with open(file_name, "r") as csvfile:
reader = csv.reader(csvfile)
# 跳过文件的第一行(表头)
next(reader)
# 创建一个字典用来存储每个学生的成绩
scores = {}
for row in reader:
stu_id, stu_name, score = row
# 如果当前学生不存在于字典中,则添加该学生
if stu_id not in scores:
scores[stu_id] = {"name": stu_name, "scores": []}
# 将该学生的成绩添加到字典中
scores[stu_id]["scores"].append(int(score))
return scores
def get_top_scores(scores):
top_scores = {}
# 遍历每个学生的成绩
for stu_id in scores:
# 获取该学生的所有成绩并排序
stu_scores = sorted(scores[stu_id]["scores"], reverse=True)
# 取出该学生的最高三次分数
top_scores[stu_id] = stu_scores[:3]
return top_scores
if __name__ == "__main__":
# 测试代码
scores = read_file("scores.txt")
top_scores = get_top_scores(scores)
for stu_id in top_scores:
print("学号:{},姓名:{},最高三次分数:{}".format(stu_id, scores[stu_id]["name"], top_scores[stu_id]))
```
上述代码中,`read_file()`函数用来读取txt文件并将每个学生的成绩存储到字典中,`get_top_scores()`函数用来找到每个学生的最高三次分数,最后在主程序中调用这两个函数并打印结果。
阅读全文
相关推荐
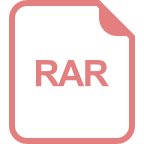
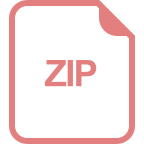
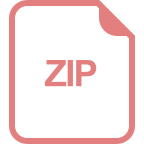




